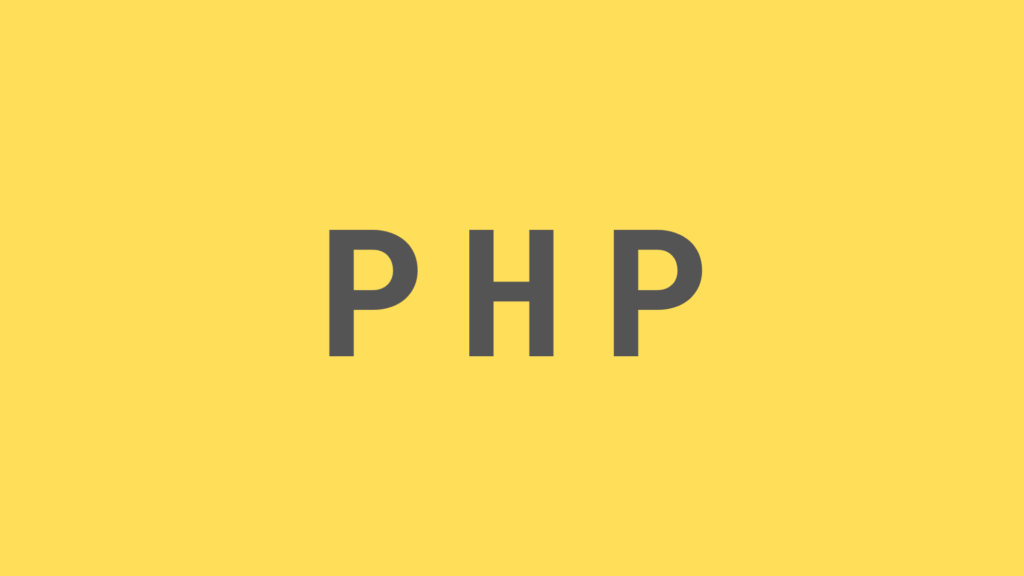
Decoding Data in PHP: The Ultimate Guide to Reading File Stream Data to String in 2025
Reading file content into a string is one of the most common tasks in PHP development. Whether you’re parsing configuration files like JSON or INI, processing uploaded documents, or consuming data from streams and APIs, being able to efficiently and correctly read file data into a string is essential.
With PHP 8.x, developers have access to mature, robust file handling functions, but choosing the right one—and understanding how to handle character encoding, memory efficiency, and errors—is key to writing performant and reliable code.
In this comprehensive guide, we’ll walk through the best ways to read file stream data into a string in PHP as of 2025, complete with modern practices, working code, and real-world insights.
Why Read File Stream Data to String in PHP?
There are many scenarios in PHP applications where you need to convert a file’s contents into a string:
- Parsing Configuration Files: Formats like JSON, INI, and YAML are typically read as strings before being parsed into arrays or objects.
- Reading Text Documents: Applications often need to display or analyze user-uploaded documents.
- Processing Network Streams: APIs or socket streams may provide data that needs to be read and handled as strings.
- General File Processing: Logging, data import/export, and command-line tools often require reading file data as text.
Methods for Reading and Converting File Stream Data to String in PHP
1. Using file_get_contents()
This is the simplest and most widely used method to read an entire file into a string.
✅ How it works:
It takes a filename (or URL) and returns the file content as a string.
📄 Code Example:
phpCopyEdit<?php
$filePath = 'data/config.json';
$content = @file_get_contents($filePath);
if ($content === false) {
echo "Error reading file.";
} else {
echo $content;
}
?>
📌 Pros:
- Very concise.
- Ideal for small to medium-sized files.
⚠️ Cons:
- Loads the entire file into memory—can be problematic with large files.
- Error handling must be explicitly added (
@
ortry/catch
via wrappers).
2. Using fread()
with fopen()
This method provides more control, allowing you to read file contents in chunks or all at once.
📄 Code Example:
phpCopyEdit<?php
$filePath = 'data/log.txt';
$handle = fopen($filePath, 'r');
if ($handle === false) {
die("Unable to open file.");
}
$content = fread($handle, filesize($filePath));
fclose($handle);
echo $content;
?>
📌 Pros:
- Greater control over how much data is read.
- Better for handling large files in chunks.
⚠️ Cons:
- Requires manual file handling.
filesize()
may not be reliable for network streams or special files.
3. Reading Line-by-Line Using fgets()
Useful when you want to process large files without loading them entirely into memory.
📄 Code Example:
phpCopyEdit<?php
$filePath = 'data/bigfile.txt';
$handle = fopen($filePath, 'r');
if ($handle === false) {
die("File open error.");
}
$content = '';
while (($line = fgets($handle)) !== false) {
$content .= $line;
}
fclose($handle);
echo $content;
?>
📌 Pros:
- Memory-efficient.
- Great for log processing or large data files.
⚠️ Cons:
- Slower than reading in one go.
- More code required to build the final string.
4. Using stream_get_contents()
Works well with generic stream resources (e.g., file streams, network connections).
📄 Code Example:
phpCopyEdit<?php
$handle = fopen('data/report.txt', 'r');
if ($handle === false) {
die("Failed to open file.");
}
$content = stream_get_contents($handle);
fclose($handle);
echo $content;
?>
📌 Pros:
- Works with open file or network streams.
- Less verbose than
fread()
in some contexts.
⚠️ Cons:
- Still reads entire file into memory.
- Not ideal for very large data sets.
5. Reading Binary Data as a String
To read raw binary data, use binary mode 'rb'
and understand the data’s encoding.
📄 Code Example:
phpCopyEdit<?php
$filePath = 'data/image.dat';
$handle = fopen($filePath, 'rb');
if (!$handle) {
die("Cannot open file.");
}
$data = fread($handle, filesize($filePath));
fclose($handle);
// Convert to string if text-based:
$decoded = mb_convert_encoding($data, 'UTF-8', 'ISO-8859-1');
echo $decoded;
?>
📌 Pros:
- Necessary for binary/text hybrids.
- Ensures data integrity with explicit encoding.
⚠️ Cons:
- You must know the original encoding.
- Risk of misinterpreting binary data as text.
Handling Character Encoding in PHP
Handling character encoding properly is crucial when working with file data, especially in multilingual or international applications.
🔧 Best Practices:
- Use UTF-8 wherever possible—it is the most compatible encoding.
- Check the encoding of files before reading using tools like
file
ormb_detect_encoding()
. - Use
mb_convert_encoding()
to convert encodings explicitly:
phpCopyEdit$content = mb_convert_encoding($content, 'UTF-8', 'ISO-8859-1');
- Set default encoding in
php.ini
:
iniCopyEditdefault_charset = "UTF-8"
- Be cautious when outputting string data to browsers or databases—set correct headers (
Content-Type: text/html; charset=UTF-8
).
Error Handling in PHP File Operations
Proper error handling ensures your application fails gracefully.
✅ Tips:
- Always check return values (
fopen()
,fread()
,file_get_contents()
). - Use
try...catch
blocks if using stream wrappers that support exceptions. - Log or report errors clearly for debugging.
📄 Basic Error Check Example:
phpCopyEdit<?php
$content = file_get_contents('data/missing.txt');
if ($content === false) {
error_log("Failed to read file: data/missing.txt");
}
?>
Best Practices for Reading File Stream Data to String in PHP
- ✅ Use
file_get_contents()
for small files and quick reads. - ✅ Use
fread()
/fgets()
for large files or when you need precise control. - ✅ Close file handles with
fclose()
to free system resources. - ✅ Check and convert character encoding as needed.
- ✅ Implement error handling using conditionals or exceptions.
- ✅ Avoid reading huge files all at once—use chunked or line-by-line methods.
- ✅ Use streams for remote sources (e.g.,
php://input
,php://memory
).
Conclusion
Reading file stream data into a string is a foundational PHP skill that underpins many applications—from file processing to configuration management and beyond. PHP 8.x offers a robust set of functions to handle this task with flexibility and precision.
Whether you’re using file_get_contents()
for quick reads, fgets()
for memory-efficient processing, or stream_get_contents()
for stream-based applications, the key is understanding the trade-offs and ensuring proper character encoding and error handling.
Mastering these techniques will help you write cleaner, safer, and more efficient PHP code—an essential skill for every modern PHP developer.