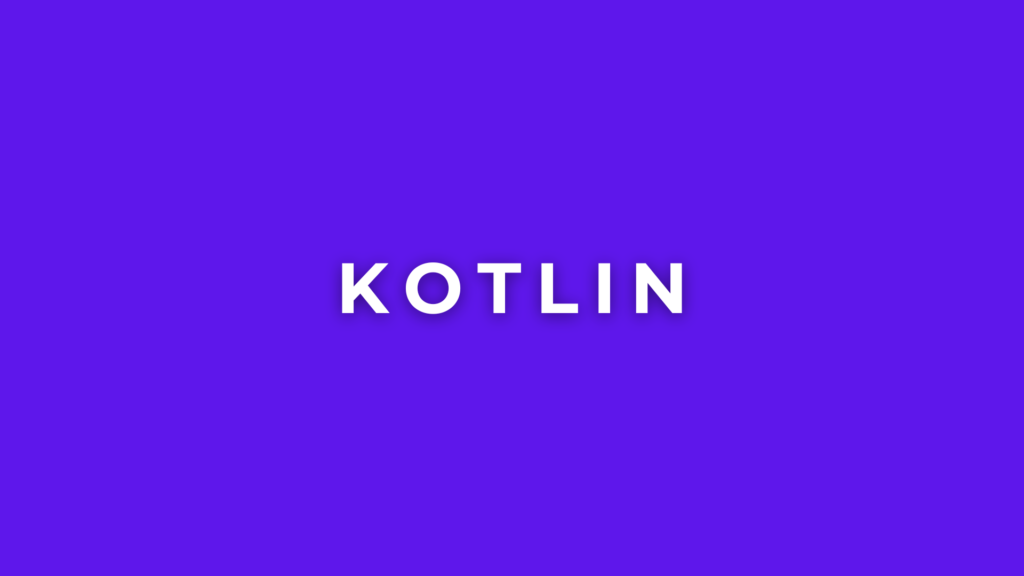
Staying Online with Kotlin: The Ultimate Guide to Checking Internet Connection in 2025
In today’s highly connected world, maintaining a seamless user experience during network fluctuations is crucial for any application. Whether you’re developing an Android app that needs to sync data or a Kotlin backend service that communicates with external APIs, reliably detecting internet connectivity is foundational to creating robust applications. This guide explores the most effective methods for checking internet connection in Kotlin applications in 2025, providing you with practical, code-based solutions for both Android and backend environments.
Why Check Internet Connection in Kotlin?
Before diving into implementation details, let’s understand why programmatically detecting network availability is essential in modern Kotlin applications:
- Preventing crashes and exceptions: Network operations can fail when offline, leading to unhandled exceptions if not properly checked.
- Enabling offline functionality: Detecting connectivity allows you to implement fallback mechanisms like caching or offline modes.
- Enhancing user experience: Proactively notifying users about connection status helps manage expectations and reduces frustration.
- Optimizing resource usage: By checking connectivity first, you can avoid unnecessary network calls that would fail anyway.
- Improving debugging and monitoring: Logging network status helps identify connectivity-related issues during development and in production.
Now, let’s explore the most reliable methods for checking internet connectivity in Kotlin applications in 2025.
Methods for Checking Internet Connection in Kotlin
1. Using InetAddress.isReachable() (Standard Kotlin/Backend)
This approach uses Java’s networking capabilities to send ICMP echo requests (similar to ping) to verify if a host is reachable.
kotlinimport java.net.InetAddress
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.withContext
/**
* Checks internet connectivity by pinging a reliable DNS server
* @param host The hostname to ping (default: Google's DNS)
* @param timeout Maximum time to wait in milliseconds
* @return true if host is reachable, false otherwise
*/
suspend fun isInternetAvailable(
host: String = "8.8.8.8",
timeout: Int = 1500
): Boolean = withContext(Dispatchers.IO) {
try {
val inetAddress = InetAddress.getByName(host)
inetAddress.isReachable(timeout)
} catch (e: Exception) {
false
}
}
Pros:
- Simple implementation that works on any Java/Kotlin platform
- Directly checks network reachability at the IP level
Cons:
- ICMP packets might be blocked by firewalls
- Requires IO operations that should be performed off the main thread
- Less reliable on Android due to potential permission issues
2. Establishing a TCP Connection with Socket (Standard Kotlin/Backend)
This method attempts to establish a TCP connection to a reliable host and port, which provides a more reliable check than ICMP.
kotlinimport java.net.InetSocketAddress
import java.net.Socket
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.withContext
/**
* Checks internet connectivity by attempting a TCP connection
* @param host The hostname to connect to
* @param port The port to connect on
* @param timeout Maximum time to wait in milliseconds
* @return true if connection succeeds, false otherwise
*/
suspend fun isOnline(
host: String = "8.8.8.8",
port: Int = 53,
timeout: Int = 1500
): Boolean = withContext(Dispatchers.IO) {
try {
Socket().use { socket ->
socket.connect(InetSocketAddress(host, port), timeout)
true
}
} catch (e: Exception) {
false
}
}
Pros:
- More reliable than ICMP as TCP connections are less likely to be blocked
- Works in both Android and backend environments
- Can be configured with different hosts for redundancy
Cons:
- Still requires IO operations that need to be performed on a background thread
- Doesn’t guarantee full internet access, only confirms TCP connectivity to specified host
3. Using ConnectivityManager on Android
Android provides platform-specific APIs through ConnectivityManager
to check network status and capabilities.
kotlinimport android.content.Context
import android.net.ConnectivityManager
import android.net.NetworkCapabilities
/**
* Checks if the device has an active internet connection
* Requires ACCESS_NETWORK_STATE permission in AndroidManifest.xml
* @param context Application or activity context
* @return true if internet is available, false otherwise
*/
fun isInternetAvailable(context: Context): Boolean {
val connectivityManager = context.getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager
val network = connectivityManager.activeNetwork ?: return false
val capabilities = connectivityManager.getNetworkCapabilities(network) ?: return false
return capabilities.hasCapability(NetworkCapabilities.NET_CAPABILITY_INTERNET) &&
capabilities.hasCapability(NetworkCapabilities.NET_CAPABILITY_VALIDATED)
}
Pros:
- Android-specific solution that provides detailed network information
- More reliable than general methods on Android devices
- Very efficient as it uses system services rather than making network calls
Cons:
- Only applicable to Android, not usable in backend Kotlin services
- Requires
ACCESS_NETWORK_STATE
permission in the manifest - Doesn’t guarantee actual internet connectivity, only reports system network status
4. Pinging a Reliable Host with HttpURLConnection
This approach makes a lightweight HTTP request to verify internet connectivity by attempting to reach a reliable web server.
kotlinimport java.net.HttpURLConnection
import java.net.URL
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.withContext
/**
* Checks internet connectivity by making a lightweight HTTP request
* @param url The URL to ping (default: Google)
* @param timeout Maximum time to wait in milliseconds
* @return true if connection succeeds, false otherwise
*/
suspend fun isOnlineWithHttp(
url: String = "https://www.google.com",
timeout: Int = 1500
): Boolean = withContext(Dispatchers.IO) {
try {
val connection = URL(url).openConnection() as HttpURLConnection
connection.apply {
connectTimeout = timeout
readTimeout = timeout
requestMethod = "HEAD" // Lightweight request, we only need headers
connect()
}
connection.responseCode in 200..299
} catch (e: Exception) {
false
}
}
Pros:
- Works across all Kotlin environments (Android and backend)
- Verifies actual HTTP connectivity, not just network availability
- Using HEAD requests minimizes data usage
Cons:
- Relies on external servers that could potentially be down
- More resource-intensive than checking local network state
- May contribute to battery drain if called frequently on mobile devices
5. Using Coroutines and try-catch for Network Requests
Rather than explicitly checking connectivity first, this pragmatic approach attempts the network operation directly within a coroutine and handles any exceptions.
kotlinimport kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.withContext
import kotlinx.coroutines.withTimeoutOrNull
import java.io.IOException
/**
* Performs a network operation with connectivity check built-in
* @param networkOperation The suspend function containing network operation
* @param timeoutMs Maximum time to wait in milliseconds
* @return Result of the operation or null if connectivity issues
*/
suspend fun <T> withConnectivityCheck(
timeoutMs: Long = 3000,
networkOperation: suspend () -> T
): Result<T> = withContext(Dispatchers.IO) {
try {
val result = withTimeoutOrNull(timeoutMs) {
networkOperation()
}
if (result != null) {
Result.success(result)
} else {
Result.failure(IOException("Operation timed out"))
}
} catch (e: Exception) {
Result.failure(e)
}
}
// Usage example
suspend fun fetchUserData(userId: String): UserData {
val result = withConnectivityCheck {
api.getUserData(userId)
}
return when {
result.isSuccess -> result.getOrThrow()
else -> {
// Handle offline case
cachedUserData(userId) ?: throw result.exceptionOrNull()!!
}
}
}
Pros:
- Idiomatic Kotlin approach using coroutines and Result type
- Combines connectivity check with the actual network operation
- Works in both Android and backend environments
Cons:
- Doesn’t explicitly check connectivity beforehand
- Relies on operation failure which might be due to reasons other than connectivity
Error Handling and Best Practices
When implementing internet connectivity checks in Kotlin applications, follow these best practices for reliable results:
- Use platform-specific APIs when available – On Android, prefer
ConnectivityManager
over generic methods. - Always implement timeouts – Network operations should never block indefinitely, especially when checking connectivity.
- Perform checks asynchronously – Use Kotlin coroutines to move network operations off the main thread.
- Consider multiple checks for redundancy – Don’t rely on a single server or method for critical applications.
- Handle state changes – On Android, register a
NetworkCallback
to be notified of connectivity changes:
kotlinimport android.net.ConnectivityManager
import android.net.Network
import android.net.NetworkCapabilities
import android.net.NetworkRequest
// Register network callback
val connectivityManager = context.getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager
val networkCallback = object : ConnectivityManager.NetworkCallback() {
override fun onAvailable(network: Network) {
// Internet connection is available
}
override fun onLost(network: Network) {
// Internet connection is lost
}
}
// Register the callback for all network types
val networkRequest = NetworkRequest.Builder()
.addCapability(NetworkCapabilities.NET_CAPABILITY_INTERNET)
.build()
connectivityManager.registerNetworkCallback(networkRequest, networkCallback)
// Don't forget to unregister when no longer needed
// connectivityManager.unregisterNetworkCallback(networkCallback)
- Be mindful of battery usage – Schedule connectivity checks appropriately, especially on mobile devices.
- Provide appropriate user feedback – Inform users about connectivity status changes with non-intrusive UI elements.
- Implement offline mode – Design your application to gracefully handle connectivity loss through caching and local storage.
Conclusion
Reliable internet connectivity detection is essential for creating robust Kotlin applications in 2025. By implementing the appropriate method for your environment—whether using platform-specific APIs like ConnectivityManager
on Android or more portable solutions like HttpURLConnection
for backend services—you can deliver a seamless user experience regardless of network conditions.
The most effective approach often combines multiple methods: using system APIs to quickly check network availability, followed by lightweight HTTP requests to verify actual internet connectivity when necessary. By following the best practices outlined in this guide and implementing proper error handling with Kotlin coroutines, you can ensure your applications remain responsive and reliable, even in challenging network environments.
Remember that connectivity checks should be part of a broader strategy for handling network operations in your Kotlin applications, including proper error handling, retry mechanisms, and offline capabilities to provide the best possible user experience.