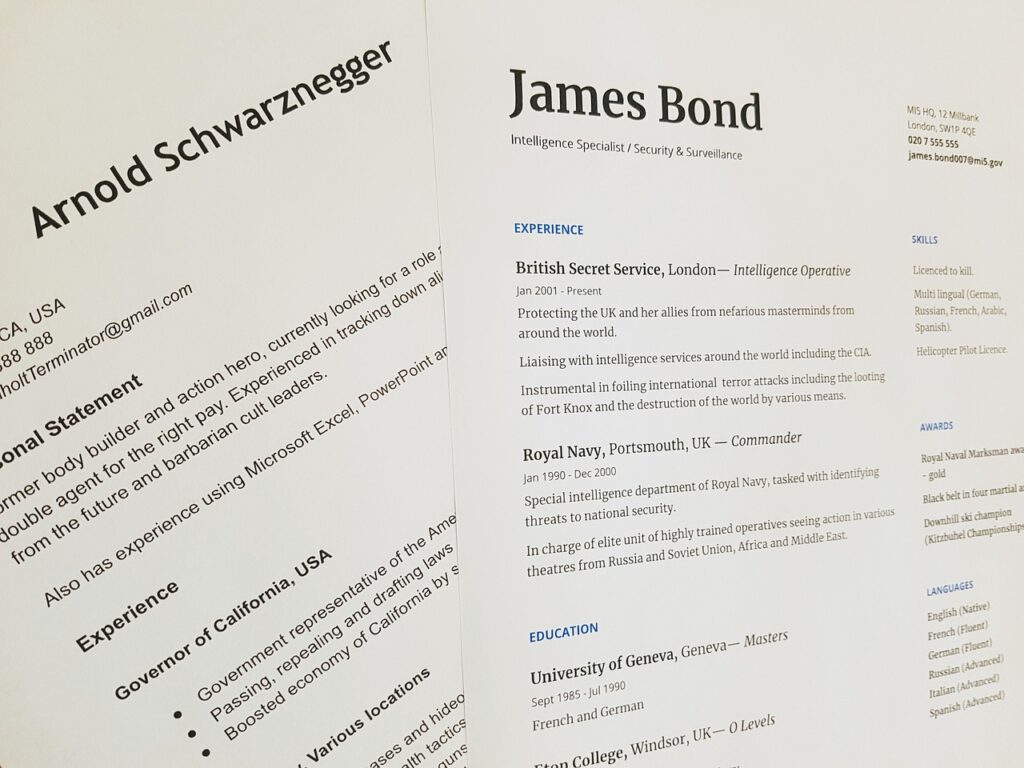
As cyber threats continue to evolve, businesses need to protect themselves from potential attacks. Penetration testing is an effective way to assess the security of a company’s IT infrastructure and identify potential vulnerabilities. However, to become a skilled penetration tester, one needs to have a diverse set of skills, including knowledge of operating systems, networking, scripting, and programming. In this article, we will discuss the essential skills needed to become a successful penetration tester and provide tips for building a strong resume that highlights your expertise.
1. Penetration Testing Techniques and Tools
As a pen testing engineer, you should have experience with various penetration testing techniques and tools, which are used to identify vulnerabilities in networks, applications, and systems. Some of the commonly used tools include:
- Metasploit: A tool used for testing and exploiting vulnerabilities in systems and applications.
- Nmap: A network exploration and port scanning tool used to discover hosts and services on a network.
- Wireshark: A network protocol analyzer used for troubleshooting, analysis, and development of communication protocols.
Other tools you may be familiar with include Burp Suite, Nessus, and Acunetix. Your experience with these tools should be highlighted on your resume, along with any relevant certifications, such as the Offensive Security Certified Professional (OSCP) or Certified Ethical Hacker (CEH).
2. Vulnerability Assessment and Remediation
In addition to using pen testing tools, a pen testing engineer should also be skilled in performing vulnerability assessments and remediation. This involves identifying potential security risks, evaluating their severity, and recommending solutions to mitigate or eliminate them.
Some of the key skills that you should possess in this area include:
- Thorough understanding of common vulnerabilities, such as SQL injection, cross-site scripting, and buffer overflows.
- Familiarity with vulnerability assessment tools, such as Nessus, OpenVAS, or Qualys.
- Ability to analyze vulnerability scan results and prioritize remediation efforts based on risk.
- Experience with implementing and testing security patches and updates.
Employers will be looking for candidates who have a proven track record of identifying and mitigating vulnerabilities, so be sure to highlight any relevant experience you have in this area on your resume.
3. Operating Systems
As a pen testing engineer, you should have experience working with various operating systems, including:
- Linux: A popular open-source operating system used in many web servers and cloud platforms.
- Windows: The dominant operating system used on desktops and servers worldwide.
- macOS: The operating system used on Apple computers and devices.
You should have a strong understanding of these operating systems, including their file systems, command-line interfaces, and networking capabilities. Additionally, you should be familiar with common security features and configurations, such as firewalls, user account management, and encryption.
4. Networking Protocols and Architectures
As a pen testing engineer, you should have a thorough understanding of networking protocols and architectures, including:
- TCP/IP: The primary protocol used for internet communication.
- HTTP/HTTPS: The protocols used for web browsing and web application communication.
- DNS: The protocol used for domain name resolution.
- VPN: Virtual private networks used for secure remote access.
- Firewalls: Network security devices used to control access to networks and systems.
You should be familiar with how these protocols and architectures work, as well as common vulnerabilities and attack vectors associated with them. Additionally, you should be able to analyze network traffic using tools such as Wireshark, and be able to identify and mitigate network-based attacks, such as denial-of-service (DoS) attacks.
Highlighting your experience with these protocols and architectures on your resume can demonstrate your proficiency in network security and your ability to identify and remediate potential vulnerabilities.
5. Web Application Security Testing
Web application security testing is a critical aspect of pen testing, and as a pen testing engineer, you should have experience with various tools and techniques used for testing web applications. Some of the skills you should possess in this area include:
- Familiarity with the OWASP Top 10, which outlines the most critical web application security risks.
- Experience with web application vulnerability scanners, such as Burp Suite, OWASP ZAP, or AppScan.
- Understanding of how web applications communicate with servers, including HTTP requests and responses, cookies, and session management.
- Ability to perform manual testing, including parameter manipulation, cross-site scripting (XSS), and SQL injection.
Your experience with web application security testing should be highlighted on your resume, along with any relevant certifications, such as the GIAC Web Application Penetration Tester (GWAPT).
6. Cloud Computing Platforms
With the increasing adoption of cloud computing, many organizations are looking for pen testing engineers who have experience with cloud platforms such as Amazon Web Services (AWS) or Microsoft Azure. Some of the skills you should possess in this area include:
- Understanding of cloud computing architectures, including virtual machines, containers, and serverless computing.
- Familiarity with cloud-specific security services, such as AWS Identity and Access Management (IAM) and Azure Active Directory.
- Ability to perform penetration testing on cloud-based environments, including virtual networks, load balancers, and storage services.
- Experience with automated cloud security testing tools, such as CloudMapper or Prowler.
Your experience with cloud computing platforms should be highlighted on your resume, along with any relevant certifications, such as the AWS Certified Security – Specialty or the Microsoft Certified: Azure Security Engineer Associate.
7. Scripting and Programming
As a pen testing engineer, you should have experience with scripting and programming languages, as these skills are essential for automating tasks and customizing tools. Some of the languages you should be familiar with include:
- Python: A versatile language used for a wide range of pen testing tasks, including scanning, exploitation, and post-exploitation.
- Bash: A command-line scripting language used for automating tasks on Linux and Unix systems.
- PowerShell: A scripting language used for automating tasks on Windows systems.
Your experience with scripting and programming should be highlighted on your resume, along with any relevant projects or contributions to open-source tools.
8. Report Writing and Communication
As a pen testing engineer, you will be expected to communicate your findings to technical and non-technical stakeholders. Therefore, you should have strong report writing and communication skills. Some of the skills you should possess in this area include:
- Ability to clearly and concisely communicate technical information to non-technical audiences.
- Experience with report writing, including creating executive summaries, technical findings, and recommendations for remediation.
- Ability to work collaboratively with other teams, such as developers, system administrators, and security analysts.
- Experience with presenting findings to clients or management.
Your experience with report writing and communication should be highlighted on your resume, along with any relevant certifications, such as the Offensive Security Certified Professional (OSCP), which requires a comprehensive report as part of the certification process.
9. Active Directory Security Testing
Active Directory (AD) is a central component of many enterprise networks, and as a pen testing engineer, you should have experience with testing its security. Some of the skills you should possess in this area include:
- Understanding of AD architecture, including domains, forests, and trusts.
- Experience with AD enumeration, including gathering information on users, groups, and computers.
- Ability to exploit AD misconfigurations, such as weak passwords or unsecured privileged accounts.
- Experience with using tools such as BloodHound, PowerView, and Mimikatz for AD security testing.
Your experience with AD security testing should be highlighted on your resume, along with any relevant certifications, such as the Microsoft Certified: Azure Security Engineer Associate or the GIAC Certified Windows Security Administrator (GCWN).
10. Mobile Application Security Testing
As mobile devices become more prevalent in the workplace, many organizations are looking for pen testing engineers who have experience with testing mobile applications. Some of the skills you should possess in this area include:
- Understanding of mobile application architecture, including the use of APIs and data storage.
- Familiarity with mobile-specific vulnerabilities, such as insecure data storage or unintended data leakage.
- Ability to perform manual testing, including intercepting and manipulating data flows.
- Experience with using mobile application security testing tools, such as Mobile Security Framework (MobSF) or Burp Suite Mobile Assistant.
Your experience with mobile application security testing should be highlighted on your resume, along with any relevant certifications, such as the Certified Mobile and IoT Penetration Tester (CMPIOT).
In conclusion, becoming a skilled penetration tester requires a lot of hard work, dedication, and a diverse set of skills. To succeed in this field, you need to stay up-to-date with the latest technologies and trends, have a strong foundation in IT fundamentals, and possess excellent communication and problem-solving skills. By mastering the essential skills outlined in this article and showcasing them in your resume, you can increase your chances of landing a job as a penetration tester and make a meaningful contribution to protecting your organization from cyber threats.