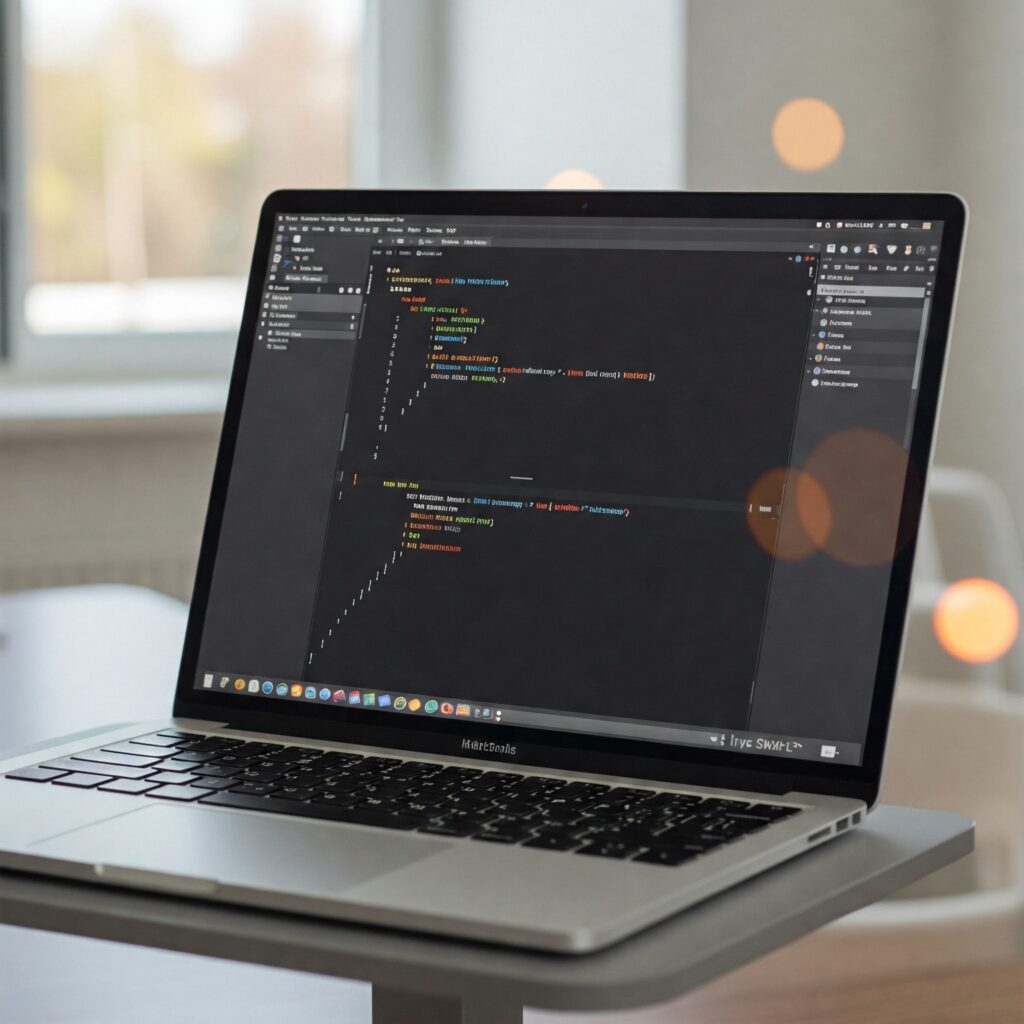
Swiftly Decoding Data: The Ultimate Guide to Reading File Stream Data to String in 2025
In today’s data-driven Swift applications, reading file content into a String
is a fundamental operation that developers perform daily. Whether you’re parsing configuration files, processing text documents, or handling data from network streams, converting file data to string representations efficiently is essential. With Swift’s continual evolution, the approaches to this common task have become more refined, offering both simplicity and performance.
This guide explores the most effective methods for reading and converting file stream data to String
in Swift 5.x, with particular attention to character encoding, error handling, and best practices for different scenarios. Let’s dive into the world of Swift file handling and unlock the most efficient ways to transform your data.
Why Read File Stream Data to String
in Swift?
Before exploring the methods, let’s understand when you might need to read file content as a String
:
- Configuration Files: Processing JSON, PLIST, YAML, or other text-based configuration formats
- Text Document Manipulation: Reading and modifying text files for content management applications
- Network Data Processing: Converting data received from API responses or web scraping
- Log File Analysis: Reading and parsing log files for debugging or monitoring
- Data Import/Export: Handling CSV files or other text-based data interchange formats
Methods for Reading and Converting File Stream Data to String
1. Using String(contentsOfFile:)
or String(contentsOf: URL)
This is the most straightforward approach when you need to read an entire file into memory at once.
do {
// Using a file path
let filePath = "/path/to/your/file.txt"
let fileContent = try String(contentsOfFile: filePath, encoding: .utf8)
// Or using a URL
let fileURL = URL(fileURLWithPath: filePath)
let fileContentFromURL = try String(contentsOf: fileURL, encoding: .utf8)
// Process the string content
print("File contains \(fileContent.count) characters")
} catch {
print("Error reading file: \(error)")
}
Advantages:
- Simple, concise syntax
- Handles encoding automatically with the specified parameter
- Ideal for small to medium-sized files
Disadvantages:
- Loads the entire file into memory at once, which can be problematic for very large files
- Will throw errors if the file doesn’t exist or the encoding is incorrect
2. Reading Line by Line with URL.lines
(Swift 5.5+)
For memory efficiency when dealing with larger files, Swift 5.5 introduced the ability to read files line by line using URL.lines
:
do {
let fileURL = URL(fileURLWithPath: "/path/to/your/file.txt")
// Process file line by line
var completeContent = ""
for try await line in fileURL.lines {
// Process each line
completeContent += line + "\n"
}
print("Processed file with content: \(completeContent)")
} catch {
print("Error processing file: \(error)")
}
Advantages:
- Memory efficient for large files
- Enables processing data incrementally
- Leverages Swift’s modern async/await syntax
Disadvantages:
- Requires joining lines if you need the complete content as one string
- Needs Swift 5.5 or later
- Must be used in an async context
3. Using FileHandle
and Data
For more granular control over file reading, especially for binary files or when you need to read specific chunks:
do {
let fileURL = URL(fileURLWithPath: "/path/to/your/file.txt")
let fileHandle = try FileHandle(forReadingFrom: fileURL)
// Modern approach (Swift 5.0+)
let data = try fileHandle.readToEnd() ?? Data()
let fileContent = String(decoding: data, as: UTF8.self)
print("File content: \(fileContent)")
// Don't forget to close the file handle
try fileHandle.close()
} catch {
print("Error reading file: \(error)")
}
For reading in chunks:
do {
let fileURL = URL(fileURLWithPath: "/path/to/your/file.txt")
let fileHandle = try FileHandle(forReadingFrom: fileURL)
// Read in chunks of 1024 bytes
let chunkSize = 1024
var fileContent = ""
while let data = try fileHandle.read(upToCount: chunkSize), !data.isEmpty {
if let chunk = String(data: data, encoding: .utf8) {
fileContent += chunk
}
}
try fileHandle.close()
print("File content read in chunks: \(fileContent)")
} catch {
print("Error reading file: \(error)")
}
Advantages:
- Fine-grained control over reading
- Memory-efficient for very large files when reading in chunks
- Works well with binary files
Disadvantages:
- More verbose code
- Requires manual handling of data conversion
- Need to manage closing the file handle
4. Reading Binary Data and Converting to String
When dealing with binary data that represents text:
do {
let fileURL = URL(fileURLWithPath: "/path/to/binary/file")
let binaryData = try Data(contentsOf: fileURL)
// Convert using specific encoding
if let content = String(data: binaryData, encoding: .utf8) {
print("Content from binary file: \(content)")
} else {
print("Failed to convert data to string - encoding might be incorrect")
}
} catch {
print("Error reading binary file: \(error)")
}
Advantages:
- Works with any data source that produces
Data
- Flexible encoding options
Disadvantages:
- Requires knowledge of the correct encoding
- Loads all data into memory at once
5. Using Scanner
for Structured Text
For structured text parsing:
do {
let fileURL = URL(fileURLWithPath: "/path/to/your/file.txt")
let fileContent = try String(contentsOf: fileURL, encoding: .utf8)
let scanner = Scanner(string: fileContent)
scanner.charactersToBeSkipped = CharacterSet.whitespaces
var parsedContent = ""
while !scanner.isAtEnd {
if let token = scanner.scanUpToCharacters(from: .newlines) {
parsedContent += "Line: \(token)\n"
}
// Skip the newline
_ = scanner.scanCharacters(from: .newlines)
}
print("Parsed content: \(parsedContent)")
} catch {
print("Error processing file: \(error)")
}
Advantages:
- Useful for parsing structured text with specific patterns
- Provides control over how content is processed
Disadvantages:
- Still requires reading the entire file first
- More complex for simple text reading tasks
Handling Character Encoding in Swift
Character encoding is crucial when reading text files. Using the wrong encoding can result in corrupted or unreadable text. Swift provides several standard encodings:
.utf8
: The most common encoding for text files and web content.ascii
: For basic ASCII text (English characters only).utf16
: Used by some systems and applications.iso2022JP
: For Japanese text.macOSRoman
: Legacy Mac encoding
Always specify the encoding explicitly when reading text files:
let content = try String(contentsOf: fileURL, encoding: .utf8)
If you’re unsure of the encoding, you might need to try multiple options:
func readWithMultipleEncodings(from url: URL) -> String? {
let encodings: [String.Encoding] = [.utf8, .ascii, .utf16, .isoLatin1]
for encoding in encodings {
if let content = try? String(contentsOf: url, encoding: encoding) {
return content
}
}
return nil
}
Error Handling
Proper error handling is essential when working with files. Swift’s do-catch
mechanism makes this straightforward:
do {
let content = try String(contentsOf: fileURL, encoding: .utf8)
// Process content
} catch let error as NSError {
switch error.code {
case NSFileReadNoSuchFileError:
print("File doesn't exist at path")
case NSFileReadInapplicableStringEncodingError:
print("Incorrect encoding specified")
default:
print("General error: \(error.localizedDescription)")
}
}
For cases where you don’t need detailed error handling, you can use try?
to get an optional result:
if let content = try? String(contentsOf: fileURL, encoding: .utf8) {
// Process content
} else {
// Handle failure
}
Best Practices
For optimal file handling in Swift 5.x:
- Choose the right method based on file size:
- Small files: Use
String(contentsOf:)
for simplicity - Large files: Use
URL.lines
or chunked reading withFileHandle
- Small files: Use
- Always specify encoding explicitly rather than relying on defaults
- Implement proper error handling using
do-catch
blocks - Close file handles when done with them
- Consider memory constraints, especially on mobile devices when reading large files
- Use async/await for file operations in Swift 5.5+ to avoid blocking the main thread
- Bundle resources require special handling:
if let bundlePath = Bundle.main.path(forResource: "config", ofType: "txt"), let content = try? String(contentsOfFile: bundlePath, encoding: .utf8) { // Process bundle resource }
Conclusion
Reading file stream data to String
in Swift has become more streamlined and efficient with modern Swift’s features. From the simple one-liners like String(contentsOf:)
to the more controlled and memory-efficient approaches using URL.lines
or chunked reading with FileHandle
, Swift provides a robust toolkit for file handling.
When choosing a method, consider your specific requirements regarding file size, memory constraints, and processing needs. Always handle character encoding correctly and implement proper error handling to ensure your file operations are reliable and robust.
By following these best practices and leveraging Swift’s modern file handling capabilities, you can efficiently transform file data into string representations for further processing in your applications.