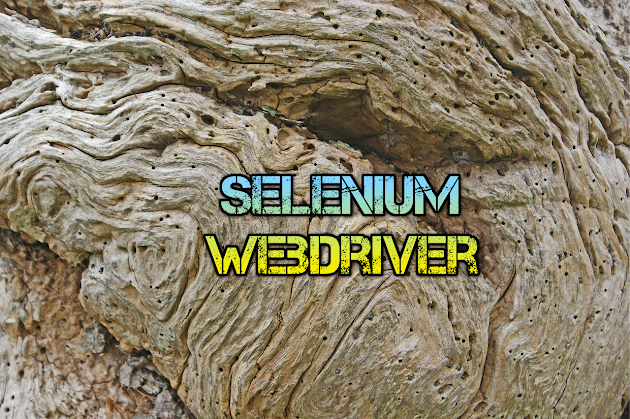
WebDriver is a open source free test automation library for doing web application testing. The main reason for extreme popularity and success of WebDriver is its ability to easily simulate the action of a real website user.
This library can control the browser and supports wide variety of browsers including all popular ones like Firefox, Chrome, Safari and IE.
The programming for web driver scripts can be done in Java, Python, Ruby and .NET. This article focuses on only Java code snippets, however the equivalent code can be easily written in any other supported language.
Some of the very common use cases like taking a error snapshot, or uploading a file are very easily accomplished using Web Driver api.
Below are some of the code snippets that I frequently use when I am creating java test cases using selenium webdriver. I hope this will help you save some time in your test case code creation.
Instantiate Specific Browser Or Client
You may need this code in every selenium script. So I thought of keeping it here handy for the popular ones.
Firefox Driver
WebDriver driver = new FirefoxDriver();
Chrome Driver
WebDriver driver = new ChromeDriver();
Safari Driver
WebDriver driver = new SafariDriver();
Internet Explorer Driver
WebDriver driver = new InternetExplorerDriver();
Android Driver
WebDriver driver = new AndroidDriver()
iPhone Driver
WebDriver driver = new IPhoneDriver();
HTML Unit
WebDriver driver = new HtmlUnitDriver()
Check If An Element Exists
You may need to perform a action based on a specific web element being present on the web page. You can use below code snippet to check if a element with id “element-id” exists on web page.
driver.findElements(By.id("element-id")).size()!=0
How To Check If An Element Is Visible With WebDriver
The above mentioned method may be good to check if a elemet exists on page. However sometimes a element may be not visible, therefore you can not perform any action on it. You can check whether an element is visible or not using below code.
WebElement element = driver.findElement(By.id("element-id")); if(element instanceof RenderedWebElement) { System.out.println("Element visible"); } else { System.out.println("Element Not visible"); }
Refresh Page
This may be required often. Just a simple refresh of the page equivalent to a browser refresh.
driver.navigate().refresh();
Navigate Back And Forward On The Browser
Navigating the history of borwser can be easily done using below two methods. The names are self explanatory.
//Go back to the last visited page driver.navigate().back(); //go forward to the next page driver.navigate().forward();
Wait For Element To Be Available
The application may load some elements late and your script needs to stop for the element to be available for next action. You can perform this check using below code.
In below code, the script is going to wait maximum 30 seconds for the element to be available. Feel free to change the maximum number per your application needs.
WebDriverWait wait = new WebDriverWait(driver, 30); WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("id123")));
Focus On A Input Element On Page
Doing focus on any element can be easily done by clicking the mouse on the required element. However when you are using selenium you may need to use this workaround instead of mouse click you can send some empty keys to a element you want to focus.
WebElement element = driver.findElement(By.id("element-id")); //Send empty message to element for setting focus on it. element.sendKeys("");
Overwrite Current Input Value On Page
The sendKeys method on WebElement class will append the value to existing value of element. If you want to clear the old value. You can use clear() method.
WebElement element = driver.findElement(By.id("element-id")); element.clear(); element.sendKeys("new input value");
Mouseover Action To Make Element Visible Then Click
When you are dealing with highly interactive multi layered menu on a page you may find this useful. In this scenario, an element is not visible unless you click on the menu bar. So below code snippet will accomplish two steps of opening a menu and selecting a menu item easily.
Actions actions = new Actions(driver); WebElement menuElement = driver.findElement(By.id("menu-element-id")); actions.moveToElement(menuElement).moveToElement(driver.findElement(By.xpath("xpath-of-menu-item-element"))).click();
Extract CSS Attribute Of An Element
This can be really helpful for getting any CSS property of a web element.
For example, to get background color of an element use below snippet
String bgcolor = driver.findElement(By.id("id123")).getCssValue("background-color");
and to get text color of an element use below snippet
String textColor = driver.findElement(By.id("id123")).getCssValue("color");
Find All Links On The Page
A simple way to extract all links from a web page.
Listlink = driver.findElements(By.tagName("a"));
Take A Screenshot On The Page
The most useful one. Selenium can capture the screenshot of any error you want to record. You may want to add this code in your exception handling logic.
File snapshot =((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
Execute A JavaScript Statement On Page
If you love JavaScript, you are going to love this. This simple JavascriptExecutor can run any javascript code snippet on browser during your testing. In case you are not able to find a way to do something using web driver, you can do that using JS easily.
Below code snippet demonstrates how you can run a alert statement on the page you are testing.
JavascriptExecutor jsx = (JavascriptExecutor) driver; jsx.executeScript("alert('hi')");
Upload A File On A Page
Uploading a file is a common use case. As of now there is not webdriver way to do this, however this can be easily done with the help of JavascriptExecutor and little bit of JS code.
String filePath = "path\to\filefor\upload"; JavascriptExecutor jsx = (JavascriptExecutor) driver; jsx.executeScript("document.getElementById('fileName').value='" + filePath + "';");
Scroll Up, Down Or Anywhere On A Page
Scrolling on any web page is required almost always. You may use below snippets to do scrolling in any direction you need.
JavascriptExecutor jsx = (JavascriptExecutor) driver; //Vertical scroll - down by 100 pixels jsx.executeScript("window.scrollBy(0,100)", ""); //Vertical scroll - up by 55 pixels (note the number is minus 55) jsx.executeScript("window.scrollBy(0,-55)", ""); //Horizontal scroll - right by 20 pixels jsx.executeScript("window.scrollBy(20,0)", ""); //Horizontal scroll - left by 95 pixels (note the number is minus 95) jsx.executeScript("window.scrollBy(-95,0)", "");
Get HTML Source Of A Element On Page
If you want to extract the HTML source of any element, you can do this by some simple Javascript code.
JavascriptExecutor jsx = (JavascriptExecutor) driver; String elementId = "element-id"; String html =(String) jsx.executeScript("return document.getElementById('" + elementId + "').innerHTML;");
Switch Between Frames In Java Using Webdriver
Multiple iframes are very common in recent web applications. You can have your webdriver script switch between different iframes easily by below code sample
WebElement frameElement = driver.findElement(By.id("id-of-frame")); driver.switchTo().frame(frameElement);
Web driver is really a powerful functional testing tool. I have not looked into any other libraries and tools after I have started using Webdriver ( selenium ) . The learning curve is really short for any java developer.
Earlier version of Selenium required a separate remote server to run the scripts. The latest version does not even require that. You can directly run a selenium webdriver script using webdriver library. This makes life of a developer even more easy.
For a quick start you may want to try the Selenium IDE. It has recording feature, that can record user actions on browser and then generate code in any supported language like Java, Python, Ruby and .NET
I hope you will like these code snippets. I have used all of them at multiple places and have them handy.
Can you think about any other common scenario that you used in web driver? Don’t forget to share with us in comments section below.
thank you good your posts in this blog برودكاست للعرب
Visibility should be checked by WebElement.isDisplayed();
With the mouse over action example, you forgot to call .perform();
The JS example isn't great, since sending an alert will block that call until the alert is dismissed (in some browsers)… doing something like .executeScript("setTimeout(function(){alert('hi');}, 1);") is a better example, then they can driver.switchTo().alert().dismiss();
Uploading a file is actually done by sending keys to the element instead. inputElement.sendKeys("/full/path/to/file");
Another way to get the html of all child elements is:
String html = driver.findElement(By.id("element-id")).getAttribute("innerHTML");
+Luke – Thanks a lot for your feedback. I will try to update these code snippets after trying your suggested code.
– Good catch on the JS alert, your idea seems much better.
– Also, I never tried the getAttribute with innerHTML, will give it a try and update the post.
The code is really useful for us !! It is totally HTML links I think due to that we can make a website !! I have some plan about the project which is related to business !! Have you any thought about that ?
I am really inspire from your thought which is encourage me to go a head !! Does web browser cause of slow network ? Thanks
how to hide the browser from opening in desktop application using selenium.
how to hide the browser from opening in desktop application using selenium.
It was nice to read your post.If you want to create robust, browser-based regression automation scale and distribute scripts across many environments then you want to use Selenium WebDriver which is a collection of language specific bindings to drive a browser. I am sure your code will definitely help many people.
for more selenium interview questions ans answers.
please visit-http://www.studyselenium.com/2013/12/100-selenium-interview-questions.html
hi Sachin,Selenium IDE can do more now – with help of SeLite. It's a framework for Selenium IDE that allows your test to access (read and write to) a test DB (isolated from the DB of the tested application).You may be even more interested in SeLite, since you use Drupal. SeLite is ideal for Drupal, because all Drupal text content is in DB. Also, Drupal 7 can work with SQLite DB, which is perfect for SeLite – so if your test deployment can use SQLite, your test data lifecycle would be very easy.See and .
Very Good…. Thanks for sharing…Please find the below useful link which provides selenium webdriver tutorials which will also guide you to build a good framework.
what is the command for scrolling down the page? so the go-to-top button will appear.
It is good but going throw full, got for the similar items , used list but not given the type of that
Ex:
List list=driver.findElements(By.id("xyz");