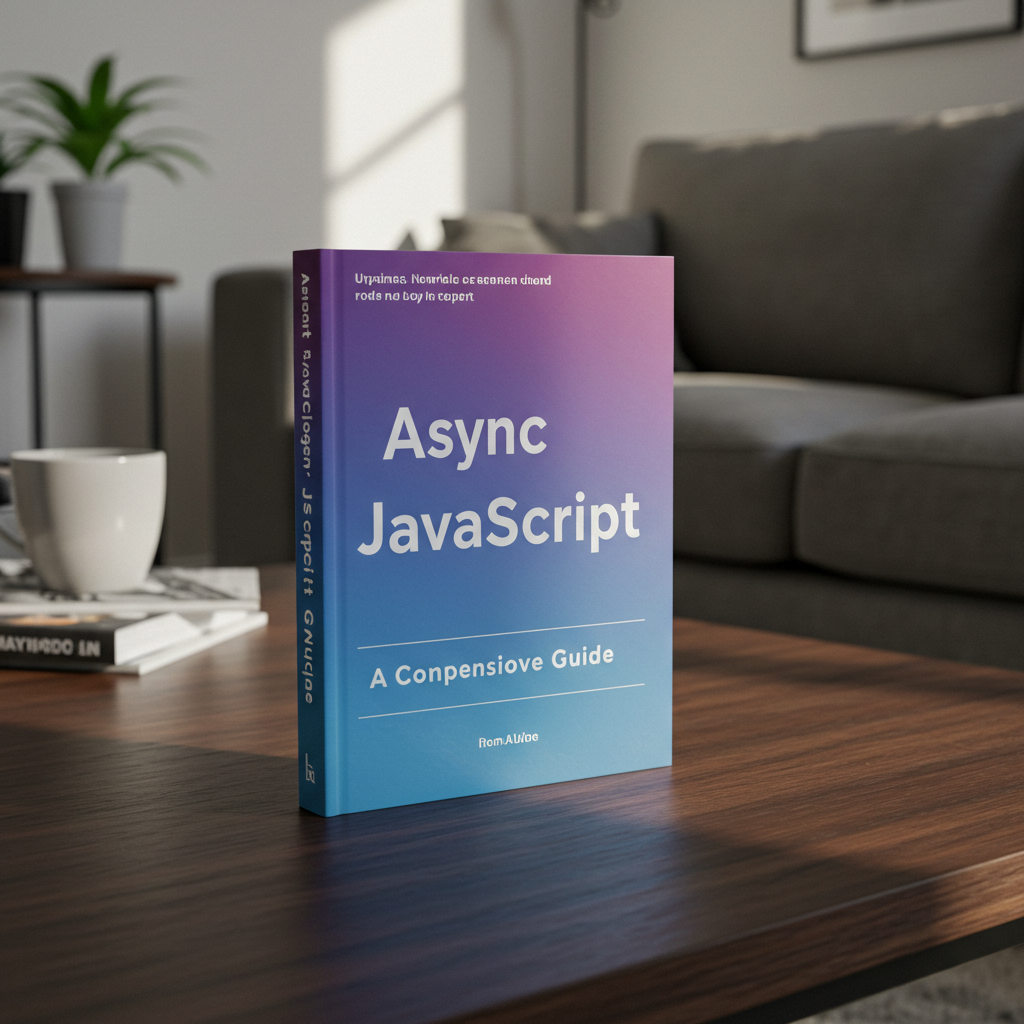
Asynchronous File Uploads in JavaScript: Methods and Best Practices
In today’s web applications, file uploading is a common requirement – from uploading profile pictures to sharing documents and media files. While traditional synchronous uploads might work for small files, they create a poor user experience by freezing the interface during the upload process. This is where asynchronous file uploads become essential.
Synchronous file uploads block the main thread, preventing users from interacting with your application until the upload completes. This can lead to frustration, especially when uploading large files or when users have slower internet connections. Asynchronous uploads, on the other hand, allow your application to remain responsive, provide real-time feedback on upload progress, and handle multiple uploads simultaneously.
This comprehensive guide will walk you through the most effective methods for implementing asynchronous file uploads in JavaScript, focusing on modern techniques like fetch
and XMLHttpRequest
. We’ll explore how to track upload progress, handle errors gracefully, and create a smooth user experience.
Understanding Asynchronous File Uploads
Asynchronous operations in JavaScript allow code execution to continue while waiting for a long-running task to complete. In the context of file uploads, this means users can continue interacting with your application while files are being transferred to the server in the background.
Benefits of Asynchronous Uploads
- Non-blocking user interface: Users can continue using your application during uploads
- Progress tracking: Real-time feedback on upload status
- Better error handling: Ability to gracefully manage and recover from upload failures
- Multiple simultaneous uploads: Upload several files concurrently
- Improved user experience: Providing feedback throughout the process reduces perceived wait time
Key JavaScript Objects for File Uploads
Before diving into implementation, let’s understand two key objects that make file uploads possible:
FormData
The FormData
interface provides a way to construct a set of key/value pairs representing form fields and their values, which can be sent using fetch
or XMLHttpRequest
. It’s particularly useful for file uploads because:
// Creating a FormData object
const formData = new FormData();
// Adding a file to the FormData object
formData.append('userFile', fileInput.files[0]);
// Adding additional form fields
formData.append('username', 'user123');
Blob
The Blob
object represents a file-like object of immutable, raw data. Blobs represent data that isn’t necessarily in a JavaScript-native format, making them perfect for handling binary data like files:
// Creating a Blob from string data
const textBlob = new Blob(['Hello, world!'], {type: 'text/plain'});
// Creating a Blob from an array buffer
const arrayBuffer = new ArrayBuffer(16);
const binaryBlob = new Blob([arrayBuffer], {type: 'application/octet-stream'});
Using fetch
for Asynchronous File Uploads
The fetch
API provides a modern, promise-based approach for making HTTP requests. While it doesn’t natively support progress events for uploads (we’ll cover a workaround later), it offers a clean and straightforward way to upload files.
Basic File Upload with fetch
Here’s how to implement a basic file upload using fetch
:
async function uploadFile(file) {
// Create FormData object
const formData = new FormData();
formData.append('file', file);
try {
// Send POST request to the server
const response = await fetch('/upload', {
method: 'POST',
body: formData
// No need to set Content-Type header - fetch sets it automatically with the boundary parameter
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const result = await response.json();
console.log('Success:', result);
return result;
} catch (error) {
console.error('Error uploading file:', error);
throw error;
}
}
// Usage
const fileInput = document.querySelector('#fileInput');
fileInput.addEventListener('change', () => {
if (fileInput.files.length > 0) {
uploadFile(fileInput.files[0]);
}
});
Uploading Multiple Files with fetch
Uploading multiple files is straightforward with FormData
:
async function uploadMultipleFiles(files) {
const formData = new FormData();
// Append multiple files with the same field name
for (let i = 0; i < files.length; i++) {
formData.append('files', files[i]);
}
try {
const response = await fetch('/upload-multiple', {
method: 'POST',
body: formData
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const result = await response.json();
console.log('Success:', result);
return result;
} catch (error) {
console.error('Error uploading files:', error);
throw error;
}
}
// Usage
const multiFileInput = document.querySelector('#multiFileInput');
multiFileInput.addEventListener('change', () => {
if (multiFileInput.files.length > 0) {
uploadMultipleFiles(multiFileInput.files);
}
});
Using XMLHttpRequest
for Asynchronous File Uploads
While fetch
is the modern approach, XMLHttpRequest
(XHR) still has advantages, particularly for its built-in progress event handling capabilities.
Basic File Upload with XMLHttpRequest
function uploadFileXHR(file) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
const formData = new FormData();
formData.append('file', file);
// Set up event listeners
xhr.upload.addEventListener('progress', (event) => {
if (event.lengthComputable) {
const percentComplete = Math.round((event.loaded / event.total) * 100);
console.log(`Upload progress: ${percentComplete}%`);
// Update UI with progress information
updateProgressBar(percentComplete);
}
});
xhr.addEventListener('load', () => {
if (xhr.status >= 200 && xhr.status < 300) {
const response = JSON.parse(xhr.responseText);
resolve(response);
} else {
reject(new Error(`HTTP error! Status: ${xhr.status}`));
}
});
xhr.addEventListener('error', () => {
reject(new Error('Network error occurred'));
});
xhr.addEventListener('abort', () => {
reject(new Error('Upload aborted'));
});
// Send the request
xhr.open('POST', '/upload', true);
xhr.send(formData);
});
}
function updateProgressBar(percentage) {
const progressBar = document.querySelector('#progressBar');
if (progressBar) {
progressBar.value = percentage;
progressBar.textContent = `${percentage}%`;
}
}
// Usage
const fileInput = document.querySelector('#fileInput');
fileInput.addEventListener('change', async () => {
if (fileInput.files.length > 0) {
try {
const result = await uploadFileXHR(fileInput.files[0]);
console.log('Upload complete:', result);
} catch (error) {
console.error('Upload failed:', error);
}
}
});
Uploading Multiple Files with XMLHttpRequest
function uploadMultipleFilesXHR(files) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
const formData = new FormData();
// Append all files
for (let i = 0; i < files.length; i++) {
formData.append('files', files[i]);
}
// Set up event listeners
xhr.upload.addEventListener('progress', (event) => {
if (event.lengthComputable) {
const percentComplete = Math.round((event.loaded / event.total) * 100);
console.log(`Upload progress: ${percentComplete}%`);
updateProgressBar(percentComplete);
}
});
xhr.addEventListener('load', () => {
if (xhr.status >= 200 && xhr.status < 300) {
const response = JSON.parse(xhr.responseText);
resolve(response);
} else {
reject(new Error(`HTTP error! Status: ${xhr.status}`));
}
});
xhr.addEventListener('error', () => {
reject(new Error('Network error occurred'));
});
xhr.addEventListener('abort', () => {
reject(new Error('Upload aborted'));
});
// Send the request
xhr.open('POST', '/upload-multiple', true);
xhr.send(formData);
});
}
Handling Progress Events
As shown in the XMLHttpRequest
examples, tracking upload progress is vital for providing feedback to users. Let’s take a deeper look at implementing progress tracking.
Creating a Progress Bar
First, let’s set up a simple HTML progress bar:
<div class="upload-container">
<progress id="progressBar" value="0" max="100">0%</progress>
<span id="progressText">0%</span>
</div>
Implementing Progress Tracking with XMLHttpRequest
function uploadWithProgress(file) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
const formData = new FormData();
formData.append('file', file);
// Progress event
xhr.upload.addEventListener('progress', (event) => {
if (event.lengthComputable) {
const percentComplete = Math.round((event.loaded / event.total) * 100);
updateUploadProgress(percentComplete);
}
});
// Other event listeners
xhr.addEventListener('load', () => {
if (xhr.status >= 200 && xhr.status < 300) {
updateUploadProgress(100); // Ensure we show 100% at the end
const response = JSON.parse(xhr.responseText);
resolve(response);
} else {
reject(new Error(`HTTP error! Status: ${xhr.status}`));
}
});
xhr.addEventListener('error', () => {
reject(new Error('Network error occurred'));
});
xhr.open('POST', '/upload', true);
xhr.send(formData);
});
}
function updateUploadProgress(percentage) {
const progressBar = document.getElementById('progressBar');
const progressText = document.getElementById('progressText');
progressBar.value = percentage;
progressText.textContent = `${percentage}%`;
// Additional visual feedback
if (percentage === 100) {
progressText.textContent = 'Upload complete!';
progressBar.classList.add('complete');
}
}
Progress Tracking with fetch
(Using Workaround)
Since fetch
doesn’t directly support progress events for uploads, we can use XMLHttpRequest
for progress tracking and then switch to fetch
for actual uploads. Alternatively, here’s a solution using the Streams API:
async function uploadWithFetchProgress(file) {
const url = '/upload';
// Create a new ReadableStream from the file
const contentLength = file.size;
let loadedBytes = 0;
const fileStream = new ReadableStream({
start(controller) {
const reader = new FileReader();
reader.onload = () => {
controller.enqueue(new Uint8Array(reader.result));
controller.close();
};
reader.onerror = (error) => controller.error(error);
reader.readAsArrayBuffer(file);
}
});
// Track the upload progress
const reportedStream = new TransformStream({
transform(chunk, controller) {
loadedBytes += chunk.length;
const percentComplete = Math.round((loadedBytes / contentLength) * 100);
updateUploadProgress(percentComplete);
controller.enqueue(chunk);
}
});
// Pipe through the progress reporter
const readableStreamClosure = fileStream.pipeThrough(reportedStream);
// Use fetch to send the stream
try {
const response = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': file.type,
'Content-Length': contentLength.toString(),
'X-File-Name': file.name
},
body: readableStreamClosure
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
updateUploadProgress(100); // Ensure we show 100% at the end
return await response.json();
} catch (error) {
console.error('Error uploading file:', error);
throw error;
}
}
Note: The Streams API may not be supported in all browsers. Check compatibility or provide fallbacks for broader support.
Error Handling and Best Practices
Proper error handling is crucial for a robust file upload system. Here are some best practices:
Comprehensive Error Handling
async function uploadWithErrorHandling(file) {
const formData = new FormData();
formData.append('file', file);
try {
// Validate file before uploading
if (!validateFile(file)) {
throw new Error('Invalid file. Please check file type and size.');
}
// Set up upload timeout
const controller = new AbortController();
const timeoutId = setTimeout(() => controller.abort(), 30000); // 30 second timeout
const response = await fetch('/upload', {
method: 'POST',
body: formData,
signal: controller.signal
});
clearTimeout(timeoutId); // Clear timeout if fetch completes
if (!response.ok) {
// Handle different error status codes
switch (response.status) {
case 413:
throw new Error('File too large. Please upload a smaller file.');
case 415:
throw new Error('Unsupported file type.');
case 401:
case 403:
throw new Error('You do not have permission to upload files.');
default:
throw new Error(`Server error: ${response.statusText}`);
}
}
return await response.json();
} catch (error) {
// Handle specific error types
if (error.name === 'AbortError') {
displayErrorMessage('Upload timed out. Please try again or check your connection.');
} else if (error.name === 'TypeError') {
displayErrorMessage('Network error. Please check your connection and try again.');
} else {
displayErrorMessage(error.message);
}
throw error;
}
}
function validateFile(file) {
// Check file size (e.g., limit to 10MB)
const maxSize = 10 * 1024 * 1024; // 10MB in bytes
if (file.size > maxSize) {
return false;
}
// Check file type (allow only specific types)
const allowedTypes = ['image/jpeg', 'image/png', 'application/pdf'];
if (!allowedTypes.includes(file.type)) {
return false;
}
return true;
}
function displayErrorMessage(message) {
const errorElement = document.getElementById('error-message');
if (errorElement) {
errorElement.textContent = message;
errorElement.style.display = 'block';
// Hide the error message after 5 seconds
setTimeout(() => {
errorElement.style.display = 'none';
}, 5000);
}
}
Security Best Practices
- File validation: Always validate file types and sizes on both client and server sides.
- Content-Type verification: Verify that the Content-Type matches the file’s actual contents.
- Rate limiting: Implement rate limiting to prevent abuse.
- Authentication and authorization: Ensure that only authorized users can upload files.
- Secure storage: Store uploaded files in a secure location with proper permissions.
- Scan for malware: If possible, scan uploaded files for malware before storing them.
- Generate new filenames: Don’t use user-provided filenames directly to prevent path traversal attacks.
User Experience Considerations
A smooth user experience makes a significant difference in how users perceive your application. Here are some key considerations:
Clear Visual Feedback
function enhancedUploadUI(files) {
const uploadContainer = document.getElementById('upload-container');
const fileList = document.getElementById('file-list');
fileList.innerHTML = ''; // Clear previous entries
// Create UI elements for each file
Array.from(files).forEach((file, index) => {
const fileItem = document.createElement('div');
fileItem.className = 'file-item';
fileItem.innerHTML = `
<div class="file-info">
<span class="file-name">${file.name}</span>
<span class="file-size">${formatFileSize(file.size)}</span>
</div>
<div class="progress-container">
<progress class="file-progress" id="progress-${index}" value="0" max="100"></progress>
<span class="progress-text" id="progress-text-${index}">0%</span>
</div>
<button class="cancel-button" id="cancel-${index}">Cancel</button>
`;
fileList.appendChild(fileItem);
// Set up cancel button
document.getElementById(`cancel-${index}`).addEventListener('click', () => {
// Cancel upload logic here
});
});
uploadContainer.classList.add('active');
}
function formatFileSize(bytes) {
if (bytes < 1024) return bytes + ' bytes';
else if (bytes < 1048576) return (bytes / 1024).toFixed(1) + ' KB';
else return (bytes / 1048576).toFixed(1) + ' MB';
}
function updateFileProgress(index, percentage) {
const progressBar = document.getElementById(`progress-${index}`);
const progressText = document.getElementById(`progress-text-${index}`);
if (progressBar && progressText) {
progressBar.value = percentage;
progressText.textContent = `${percentage}%`;
if (percentage === 100) {
progressText.textContent = 'Complete';
progressBar.classList.add('complete');
}
}
}
Handling Large File Uploads
For large files, consider these approaches:
- Chunked uploads: Split large files into smaller chunks and upload them sequentially or in parallel.
- Resumable uploads: Allow users to resume interrupted uploads.
- File compression: Compress files before uploading when appropriate.
Here’s an example of a chunked upload implementation:
async function uploadLargeFile(file) {
const chunkSize = 1024 * 1024; // 1MB chunks
const totalChunks = Math.ceil(file.size / chunkSize);
let uploadedChunks = 0;
const fileId = generateUniqueId(); // Generate a unique ID for this file
for (let start = 0; start < file.size; start += chunkSize) {
const chunk = file.slice(start, start + chunkSize);
const formData = new FormData();
formData.append('chunk', chunk);
formData.append('fileId', fileId);
formData.append('chunkIndex', Math.floor(start / chunkSize));
formData.append('totalChunks', totalChunks);
formData.append('fileName', file.name);
try {
const response = await fetch('/upload-chunk', {
method: 'POST',
body: formData
});
if (!response.ok) {
throw new Error(`Failed to upload chunk ${Math.floor(start / chunkSize) + 1}/${totalChunks}`);
}
uploadedChunks++;
const percentComplete = Math.round((uploadedChunks / totalChunks) * 100);
updateUploadProgress(percentComplete);
} catch (error) {
console.error('Chunk upload failed:', error);
throw error;
}
}
// Notify server that all chunks have been uploaded
try {
const response = await fetch('/complete-upload', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
fileId,
fileName: file.name,
totalChunks
})
});
if (!response.ok) {
throw new Error('Failed to complete the upload');
}
return await response.json();
} catch (error) {
console.error('Failed to complete upload:', error);
throw error;
}
}
function generateUniqueId() {
return Date.now().toString(36) + Math.random().toString(36).substr(2, 5);
}
Server-Side Considerations
While this article focuses on client-side implementation, it’s important to understand how server-side handling works.
Common Server Technologies
- Node.js with Express: Uses
multer
middleware for handling file uploads - PHP: Uses
$_FILES
superglobal withmove_uploaded_file()
- Python with Flask/Django: Uses various extensions like
flask-uploads
or Django’sFileField
- Java with Spring: Uses
MultipartFile
interface - .NET Core: Uses
IFormFile
interface
Server-Side Example (Node.js with Express)
const express = require('express');
const multer = require('multer');
const path = require('path');
const fs = require('fs');
const app = express();
// Set up storage for uploaded files
const storage = multer.diskStorage({
destination: (req, file, cb) => {
cb(null, 'uploads/');
},
filename: (req, file, cb) => {
// Generate safe filename
const uniqueSuffix = Date.now() + '-' + Math.round(Math.random() * 1E9);
cb(null, uniqueSuffix + path.extname(file.originalname));
}
});
// File filter function
const fileFilter = (req, file, cb) => {
// Accept images and PDFs only
if (file.mimetype.startsWith('image/') || file.mimetype === 'application/pdf') {
cb(null, true);
} else {
cb(new Error('Unsupported file type'), false);
}
};
// Create the multer instance
const upload = multer({
storage: storage,
limits: {
fileSize: 10 * 1024 * 1024, // 10MB
files: 5 // Max 5 files at once
},
fileFilter: fileFilter
});
// Single file upload endpoint
app.post('/upload', upload.single('file'), (req, res) => {
try {
if (!req.file) {
return res.status(400).json({ error: 'No file uploaded' });
}
// Process the file - in a real app, you might save info to a database
return res.status(200).json({
message: 'File uploaded successfully',
fileDetails: {
filename: req.file.filename,
size: req.file.size,
mimetype: req.file.mimetype
}
});
} catch (error) {
console.error('Error uploading file:', error);
return res.status(500).json({ error: 'File upload failed' });
}
});
// Multiple files upload endpoint
app.post('/upload-multiple', upload.array('files', 5), (req, res) => {
try {
if (!req.files || req.files.length === 0) {
return res.status(400).json({ error: 'No files uploaded' });
}
const filesDetails = req.files.map(file => ({
filename: file.filename,
size: file.size,
mimetype: file.mimetype
}));
return res.status(200).json({
message: 'Files uploaded successfully',
count: req.files.length,
files: filesDetails
});
} catch (error) {
console.error('Error uploading files:', error);
return res.status(500).json({ error: 'File upload failed' });
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Conclusion
Asynchronous file uploads are essential for creating responsive, user-friendly web applications. By implementing the methods and best practices covered in this guide, you can provide your users with a smooth and informative upload experience.
Key takeaways from this article:
- Use
FormData
objects to prepare files for upload - Choose the right upload method based on your needs (
fetch
for simplicity,XMLHttpRequest
for progress tracking) - Always provide visual feedback on upload progress
- Implement comprehensive error handling
- Consider user experience for large file uploads
- Ensure server-side validation and security
Remember that file uploads often involve sensitive data, so always prioritize security and validation both on the client and server sides. Test your implementation thoroughly across different browsers and connection speeds to ensure a consistently good experience for all users.
By following these guidelines, you’ll be well-equipped to implement robust asynchronous file upload functionality in your JavaScript applications.