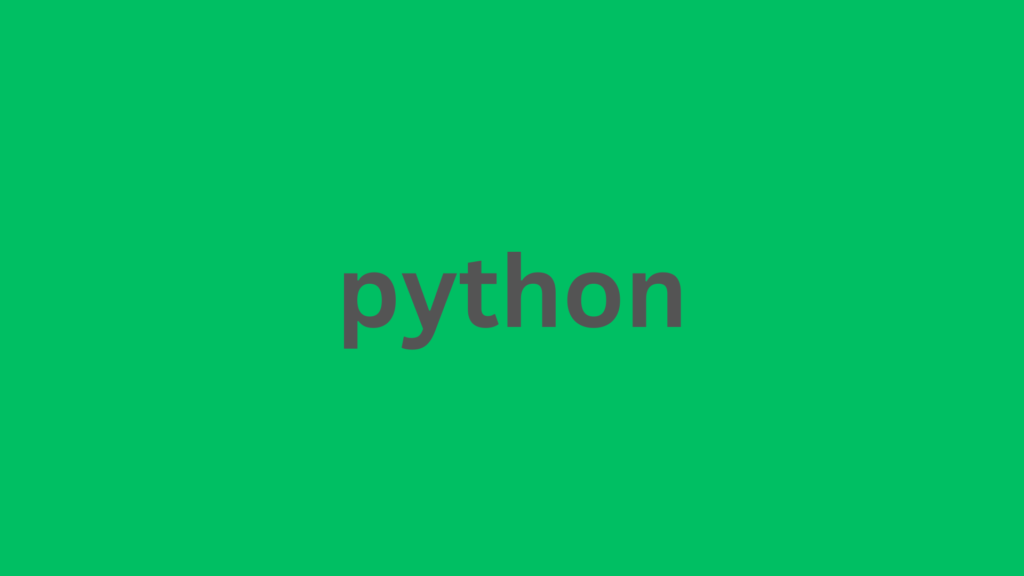
Python is a popular and powerful programming language that provides various functionalities for working with dates and time. One of the common tasks in working with dates is to create instances of past or future dates. Python’s built-in datetime
module provides several ways to accomplish this. In this article, we will explore how to get an instance of a past or future date in Python.
How To Get Instance Of Past Date?
To get an instance of a past date in Python, you can use the datetime
module. Here’s an example of how to create an instance of a date that represents one week ago from the current date:
import datetime today = datetime.date.today() one_week_ago = today - datetime.timedelta(days=7) print(one_week_ago)
Output
2023-03-19
In the example above, we first get the current date using datetime.date.today()
, and then subtract a datetime.timedelta
object representing 7 days from it using the -
operator. The resulting one_week_ago
variable is an instance of the date
class representing the date that was one week ago from the current date.
How To Get Instance Of Future Date?
To get an instance of a future date in Python, you can also use the datetime
module. Here’s an example of how to create an instance of a date that represents one week from today:
import datetime today = datetime.date.today() one_week_from_today = today + datetime.timedelta(days=7) print(one_week_from_today)
Output:
2023-04-02
In the example above, we first get the current date using datetime.date.today()
, and then add a datetime.timedelta
object representing 7 days to it using the +
operator. The resulting one_week_from_today
variable is an instance of the date
class representing the date that is one week from today’s date.
Working with dates is a common task in many programming applications, and Python’s datetime
module provides an easy way to create instances of past or future dates. Whether you need to create a date instance that represents a week ago or a date instance that is a month from today, Python’s datetime
module provides a simple and efficient way to accomplish these tasks. By using the techniques we have discussed in this article, you can easily work with dates in your Python applications.