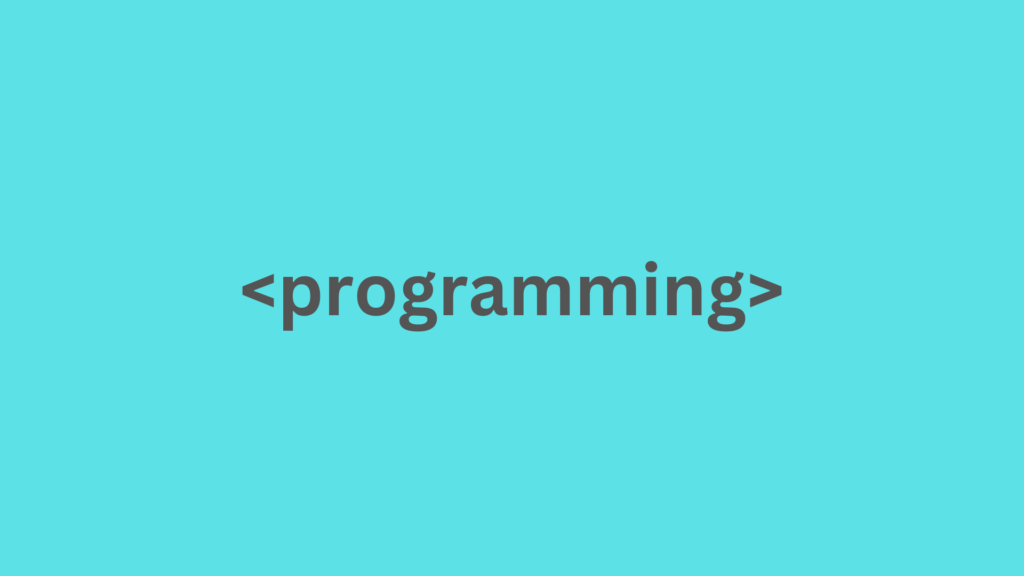
In JavaScript, arrays are commonly used to store a collection of values. There may be situations where you need to remove a specific item from an array. Fortunately, there are several ways to achieve this in JavaScript. In this article, we will explore two common ways to remove a specific item from an array: using the splice()
method and the filter()
method.
Using IndexOf and Splice Method To Remove an Element
In JavaScript, you can remove a specific item from an array using the splice()
method. The splice()
method takes two arguments: the index of the item to remove and the number of items to remove.
Here is an example code snippet that removes the item with value 2
from an array:
let array = [1, 2, 3, 4, 5]; let index = array.indexOf(2); // Get the index of the item to remove if (index !== -1) { // If the item exists in the array array.splice(index, 1); // Remove the item } console.log(array); // Output: [1, 3, 4, 5]
In the above code, indexOf()
is used to get the index of the item to remove. If the item is not found in the array, indexOf()
returns -1
.
The if
statement is used to check if the item exists in the array. If the item exists, the splice()
method is called with the index of the item to remove and the number 1
, which indicates that one item should be removed.
Using Filter Method To Remove an Element
In JavaScript, you can also remove a specific item from an array using the filter()
method. The filter()
method creates a new array with all elements that pass the test implemented by the provided function.
Here is an example code snippet that removes the item with value 2
from an array using filter()
:
let array = [1, 2, 3, 4, 5];
array = array.filter(function(item) {
return item !== 2;
});
console.log(array); // Output: [1, 3, 4, 5]
In the above code, filter()
method creates a new array that includes all items except the item with value 2
. The provided function checks if each item is not equal to 2
using the strict not equal (!==
) operator. If the item is not equal to 2
, the function returns true
, and the item is included in the new array. If the item is equal to 2
, the function returns false
, and the item is excluded from the new array.
Removing a specific item from an array is a common operation in JavaScript. In this article, we have discussed two approaches to remove an item from an array using the splice()
and filter()
methods. The splice()
method modifies the original array by removing the item at a specified index, while the filter()
method returns a new array that excludes the specified item. Both methods have their advantages and disadvantages depending on the specific use case. By understanding these techniques, you can effectively remove specific items from an array in JavaScript.