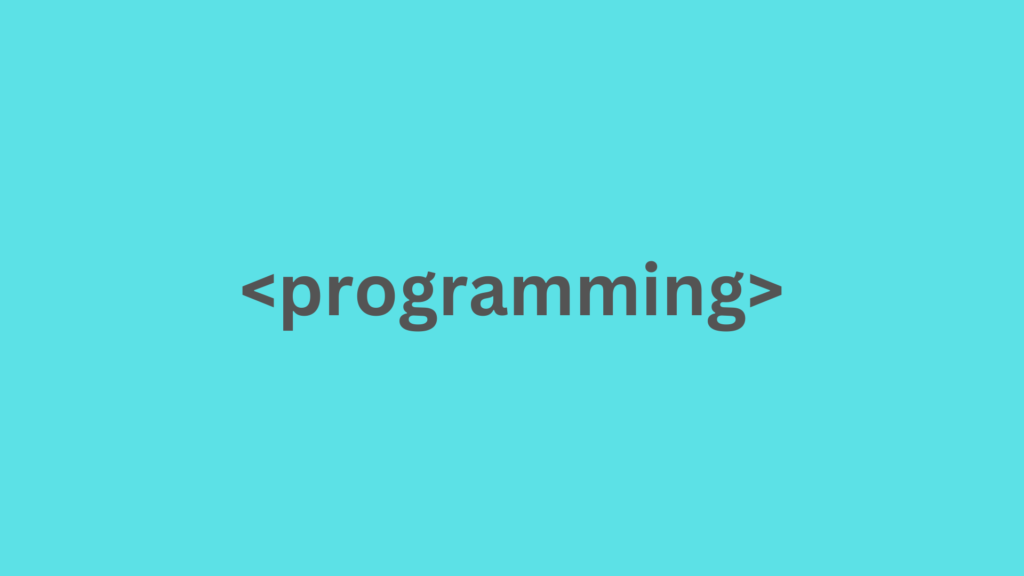
Extracting file extensions from filenames is a common task in programming, especially in file management and processing applications. In Python, you can use the os
module to extract the file extension from a filename. By knowing how to extract the file extension, you can process and manipulate files based on their types and ensure that your program handles different file formats correctly.
In Python, you can extract the file extension from a filename using the os
module. Here’s an example code snippet:
import os filename = "example.txt" file_extension = os.path.splitext(filename)[1] print(file_extension) # Output: ".txt"
The os.path.splitext()
method separates the filename into two parts – the root and the extension – and returns them as a tuple. In this case, we’re interested in the second element of the tuple, which contains the file extension.
If the filename doesn’t have an extension, os.path.splitext()
will return an empty string as the second element of the tuple. For example:
import os
filename = "example"
file_extension = os.path.splitext(filename)[1]
print(file_extension) # Output: ""
In this case, file_extension
will be an empty string.
The syntax used to extract the file extension from a filename using the os
module should be applicable to all versions of Python, as the os
module is a built-in module that is available in all Python versions.
Extracting file extensions from filenames is an important task for managing and processing files in Python. By using the os
module, you can extract the file extension from a filename and use it to perform specific operations on files based on their types. With this knowledge, you can write more robust and efficient programs that can handle different file formats with ease.