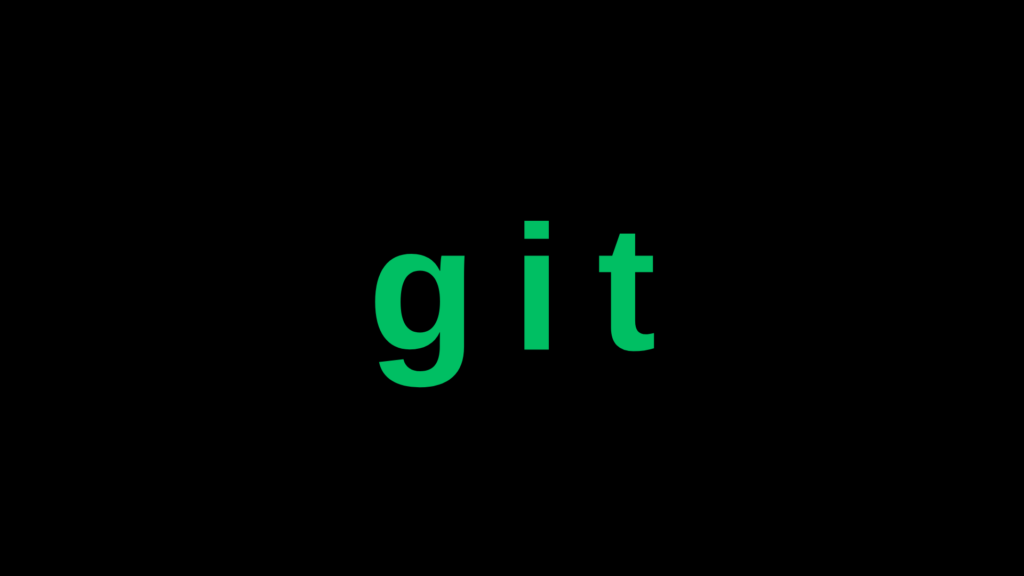
Essential Git Commands Every Developer Should Know: A Practical Guide
In the fast-paced world of software development, version control is no longer optional—it’s essential. Git has emerged as the industry standard for version control, empowering developers to track changes, collaborate seamlessly, and maintain code integrity throughout the development lifecycle. Whether you’re working solo or as part of a large team, mastering Git commands is crucial for efficient workflow management and effective collaboration.
This practical guide aims to equip developers of all experience levels with the essential Git commands needed for everyday version control tasks. By understanding these fundamental commands and their applications, you’ll improve your productivity, reduce errors, and enhance your ability to collaborate with other developers.
Understanding Git Basics
Before diving into specific commands, let’s briefly review the core concepts that form Git’s foundation:
Key Git Concepts
- Repository (Repo): A directory containing your project’s files and the entire history of changes made to those files.
- Commit: A snapshot of your repository at a specific point in time, including a unique identifier and a message describing the changes.
- Branch: An independent line of development that allows you to work on features or fixes without affecting the main codebase.
- Merge: The process of integrating changes from one branch into another.
- Remote: A version of your repository hosted on a server, enabling collaboration with other developers.
Git Workflow Stages
Git operates through a three-stage workflow:
- Working Directory: Where you make changes to your files.
- Staging Area (Index): Where you prepare changes for committing by selecting which modifications to include.
- Repository: Where Git permanently stores changes as commits.
Understanding this workflow is critical as we explore the commands that manage the movement of code through these stages.
Essential Git Commands
Repository Setup and Configuration
git init
The git init
command creates a new Git repository in your current directory, establishing the necessary file structure for Git to begin tracking changes.
Example:
mkdir my-new-project
cd my-new-project
git init
Best Practices:
- Initialize Git at the root of your project directory.
- Create a
.gitignore
file immediately after initialization. - Don’t create repositories inside other Git repositories.
Common Error: Running git init
in an existing repository will reinitialize it. This isn’t harmful but might be unintended.
git clone
The git clone
command creates a copy of an existing repository, including all files, branches, and commit history.
Example:
git clone https://github.com/username/repository-name.git
Options:
- Clone a specific branch:
git clone -b branch-name repository-url
- Create a custom directory name:
git clone repository-url custom-folder-name
Best Practice: When working with large repositories, consider using a shallow clone to save bandwidth and disk space: git clone --depth=1 repository-url
Basic File Operations
git add
The git add
command moves changes from your working directory to the staging area, preparing them for a commit.
Examples:
# Add a specific file
git add filename.js
# Add multiple files
git add file1.js file2.js
# Add all modified files
git add .
# Add all files with specific extension
git add *.js
# Add files interactively
git add -p
Best Practices:
- Review changes before adding them using
git status
orgit diff
. - Add related changes together for cohesive commits.
- Use
git add -p
to review and stage changes in chunks.
Common Error: Adding files unintentionally. Use git reset filename
to unstage.
git commit
The git commit
command records the staged changes in the repository history, creating a new commit with a unique identifier.
Examples:
# Commit with a message
git commit -m "Add user authentication feature"
# Add all tracked files and commit in one step
git commit -am "Fix navigation bar styling"
Best Practices:
- Write clear, concise commit messages that explain the “why” behind changes.
- Follow conventional commit message formats (e.g., “feat: add user authentication”).
- Keep commits focused on a single logical change.
- Commit frequently to create a detailed history.
Common Error: Forgetting to stage files before committing. Use git status
to verify staged files.
git status
The git status
command displays the state of your working directory and staging area, showing which files are modified, staged, or untracked.
Example:
git status
Best Practices:
- Run
git status
frequently to stay informed about your repository’s state. - Use the
-s
flag for a more concise output:git status -s
git log
The git log
command displays the commit history, showing commit messages, authors, dates, and commit IDs.
Examples:
# Basic log
git log
# Compact log format
git log --oneline
# Show log with graph visualization
git log --graph --oneline --decorate
# Show changes in each commit
git log -p
# Show last N commits
git log -n 5
Best Practices:
- Use
git log --oneline
for a quick overview. - Filter logs with
git log --author="username"
orgit log --since="2023-01-01"
. - Create custom aliases for frequently used log formats.
git diff
The git diff
command shows changes between commits, the working directory, and the staging area.
Examples:
# Show unstaged changes
git diff
# Show staged changes
git diff --staged
# Compare two commits
git diff commit1..commit2
# Compare a file between commits
git diff commit1..commit2 -- filename
Best Practices:
- Review changes with
git diff
before staging them. - Use a tool like
git difftool
for a more user-friendly visualization.
Branch Management
git branch
The git branch
command lists, creates, or deletes branches in your repository.
Examples:
# List all local branches
git branch
# List all branches (local and remote)
git branch -a
# Create a new branch
git branch feature-login
# Delete a branch
git branch -d feature-login
# Force delete a branch
git branch -D feature-login
Best Practices:
- Use descriptive branch names prefixed with a category (e.g.,
feature/
,bugfix/
,hotfix/
). - Regularly clean up merged branches.
- Don’t delete branches that haven’t been merged unless certain.
Common Error: Deleting branches that contain unique work. Use -d
(which checks for unmerged work) before resorting to -D
.
git checkout
The git checkout
command switches between branches or restores working tree files.
Examples:
# Switch to an existing branch
git checkout develop
# Create and switch to a new branch
git checkout -b feature-signup
# Discard changes in a file
git checkout -- filename.js
Best Practices:
- Ensure your working directory is clean before switching branches.
- Use
git checkout -b
to create and switch to a branch in one command. - Consider using the newer
git switch
andgit restore
commands which provide more explicit functionality.
Common Error: Losing uncommitted changes when switching branches. Commit, stash, or discard changes first.
git merge
The git merge
command integrates changes from one branch into another.
Examples:
# Merge a feature branch into current branch
git merge feature-login
# Merge with commit (even if fast-forward is possible)
git merge --no-ff feature-login
# Abort a merge in progress
git merge --abort
Best Practices:
- Ensure your working directory is clean before merging.
- Update your base branch (e.g.,
main
) before merging. - Use
--no-ff
for feature branches to maintain history context. - Test your code after merging to catch any conflicts that may have been missed.
Common Error: Merge conflicts. Resolve them by editing the conflicted files, then git add
and git commit
.
Remote Operations
git pull
The git pull
command fetches changes from a remote repository and integrates them into the current branch.
Examples:
# Pull from default remote (origin)
git pull
# Pull from specific remote and branch
git pull origin develop
# Pull with rebase instead of merge
git pull --rebase origin main
Best Practices:
- Use
git pull --rebase
to maintain a cleaner history when appropriate. - Always pull before pushing to avoid unnecessary conflicts.
- Consider using
git fetch
followed bygit merge
for more control.
Common Error: Conflicts during pull. Resolve conflicts, then continue with git add
and git commit
or git rebase --continue
.
git push
The git push
command sends local commits to a remote repository.
Examples:
# Push to default remote and branch
git push
# Push to specific remote and branch
git push origin feature-branch
# Push and set upstream branch
git push -u origin feature-branch
# Force push (use with caution!)
git push --force
Best Practices:
- Always pull before pushing to avoid conflicts.
- Use
-u
flag when pushing a new branch to set the upstream. - Avoid force pushing to shared branches; it can overwrite others’ work.
Common Error: Push rejection due to non-fast-forward updates. Pull first, resolve conflicts if any, then push again.
git remote
The git remote
command manages connections to remote repositories.
Examples:
# List remote repositories
git remote -v
# Add a new remote
git remote add upstream https://github.com/original-repo/project.git
# Remove a remote
git remote remove upstream
# Rename a remote
git remote rename origin github
Best Practices:
- Use descriptive names for remotes (e.g.,
origin
for your fork,upstream
for the original repository). - Regularly verify your remote URLs with
git remote -v
.
Undoing Changes
git reset
The git reset
command undoes changes by moving the HEAD and branch pointer to a specified commit.
Examples:
# Unstage changes but keep them in working directory
git reset HEAD filename.js
# Undo the last commit but keep changes staged
git reset --soft HEAD~1
# Undo the last commit and unstage changes
git reset HEAD~1
# Undo the last commit and discard changes (dangerous!)
git reset --hard HEAD~1
Best Practices:
- Use
--soft
or default reset when you want to keep your changes. - Be extremely cautious with
--hard
reset as it permanently discards changes. - Consider
git revert
for commits that have already been pushed.
Common Error: Unintended data loss with --hard
reset. There’s no easy recovery, so use with caution.
git revert
The git revert
command creates a new commit that undoes the changes made in a previous commit.
Examples:
# Revert the most recent commit
git revert HEAD
# Revert a specific commit
git revert commit-hash
# Revert multiple commits
git revert commit1..commit3
Best Practices:
- Use
git revert
instead ofgit reset
for commits that have been pushed to a shared repository. - Review the changes that will be reverted before confirming.
Common Error: Revert conflicts. Resolve conflicts manually, then continue with git add
and git revert --continue
.
Temporary Storage
git stash
The git stash
command temporarily saves modified, tracked files to work on something else without committing incomplete work.
Examples:
# Stash current changes
git stash
# Stash with a descriptive message
git stash save "Work in progress on login feature"
# List all stashes
git stash list
# Apply the most recent stash
git stash apply
# Apply a specific stash
git stash apply stash@{2}
# Apply and remove stash
git stash pop
# Remove a stash
git stash drop stash@{1}
# Clear all stashes
git stash clear
Best Practices:
- Always provide a descriptive message with
git stash save "message"
. - Use
git stash list
to keep track of your stashes. - Clean up stashes with
git stash drop
when no longer needed.
Common Error: Forgetting stashed changes. Regularly review your stash list and clean up unnecessary stashes.
Configuration and Ignore Rules
.gitignore
The .gitignore
file specifies intentionally untracked files that Git should ignore.
Example .gitignore file:
# Node.js dependencies
node_modules/
# Build outputs
dist/
build/
# Environment variables
.env
.env.local
# IDE files
.vscode/
.idea/
# Logs
*.log
npm-debug.log*
# Operating system files
.DS_Store
Thumbs.db
Best Practices:
- Create a
.gitignore
file at the beginning of your project. - Include environment-specific files, build artifacts, and dependency directories.
- Use patterns like
*.log
to ignore all files with specific extensions. - Consider using global
.gitignore
files for personal preferences (e.g., IDE files).
Common Error: Tracking files that should be ignored. If a file is already tracked, simply adding it to .gitignore
won’t stop tracking it. Use git rm --cached filename
first.
Git Workflow Best Practices
Effective Branching Strategies
GitFlow Workflow
The GitFlow workflow defines a strict branching model designed around project releases:
- main/master: Contains production-ready code
- develop: Integration branch for features
- feature/: Individual feature branches
- release/: Preparation for a new production release
- hotfix/: Urgent fixes for production issues
This strategy works well for projects with scheduled release cycles.
Feature Branch Workflow
A simpler approach where all feature development takes place in dedicated branches:
- Create a branch from main for each feature
- Develop the feature in isolation
- Submit a pull request for code review
- Merge back to main when approved
This strategy is flexible and works well for continuous delivery environments.
Writing Effective Commit Messages
Clear commit messages are crucial for maintaining a useful Git history:
- Use a structured format:
<type>: <short summary> <detailed description>
Where<type>
can be:- feat: A new feature
- fix: A bug fix
- docs: Documentation changes
- style: Formatting changes
- refactor: Code restructuring
- test: Test additions or modifications
- chore: Maintenance tasks
- Keep the subject line concise (50 characters or less)
- Use imperative mood (“Add feature” not “Added feature”)
- Explain the why, not just the what in the description
Resolving Merge Conflicts
Merge conflicts occur when Git can’t automatically merge changes. To resolve them:
- Run
git status
to identify conflicted files - Open each conflicted file and look for conflict markers (
<<<<<<<
,=======
,>>>>>>>
) - Edit the files to resolve conflicts
- Use
git add
to mark conflicts as resolved - Complete the merge with
git commit
Prevention Tips:
- Pull frequently to minimize divergence
- Communicate with team members about which files you’re working on
- Break large features into smaller, focused commits
- Use feature flags to integrate incomplete features safely
Common Git Scenarios and Solutions
Undoing Unintended Commits
Scenario 1: You just committed but haven’t pushed yet
# Undo commit but keep changes staged
git reset --soft HEAD~1
# Undo commit and unstage changes
git reset HEAD~1
Scenario 2: You’ve already pushed the commit
# Create a new commit that undoes the previous one
git revert HEAD
git push
Recovering Deleted Files
Scenario 1: Files deleted but not committed
# Restore file from the index
git checkout -- deleted_file.txt
Scenario 2: Files deleted and committed but not pushed
# Find the commit where the file was deleted
git log -- deleted_file.txt
# Reset to the commit before deletion
git reset HEAD~1
# Or checkout just that file from an earlier commit
git checkout commit_hash -- deleted_file.txt
Cleaning Up Local Branches
Scenario: Removing branches that have been merged
# List merged branches
git branch --merged
# Delete a merged branch
git branch -d feature-branch
# Delete all merged branches except main and develop
git branch --merged | grep -v "\*\|main\|develop" | xargs git branch -d
Finding the Source of a Bug
Scenario: Identifying which commit introduced a bug
# Start the binary search process
git bisect start
# Mark the current commit as bad
git bisect bad
# Mark a known good commit
git bisect good commit_hash
# Git will checkout commits for you to test
# After testing, mark each as good or bad
git bisect good # or git bisect bad
# When the process completes, git will identify the first bad commit
# Return to your original branch
git bisect reset
Conclusion
Mastering these essential Git commands will significantly enhance your development workflow and collaboration abilities. From basic operations like committing changes to more advanced techniques like resolving merge conflicts and tracking down bugs, Git provides powerful tools for managing your codebase throughout its lifecycle.
Remember that Git proficiency comes with practice. Start by incorporating these commands into your daily workflow, gradually expanding your repertoire as you become more comfortable. Make use of Git’s built-in help system (git help command
) when you need more information about specific commands.
To deepen your Git knowledge, consider exploring more advanced topics like rebasing, cherry-picking, and Git hooks. The official Git documentation is an excellent resource for continued learning, as are platforms like GitHub Learning Lab and Atlassian’s Git tutorials.
By investing time in learning Git commands now, you’re setting yourself up for more efficient collaboration, cleaner code history, and fewer version control headaches in your development journey.
Meta Data Suggestions:
- Title: “Essential Git Commands Every Developer Should Know: A Practical Guide”
- Description: “Master version control with this comprehensive guide to essential Git commands, workflows, and best practices for modern software development.”
- Keywords: Git commands, version control, git tutorial, git workflow, essential git commands, git branch, git merge, git push, git pull, git basics