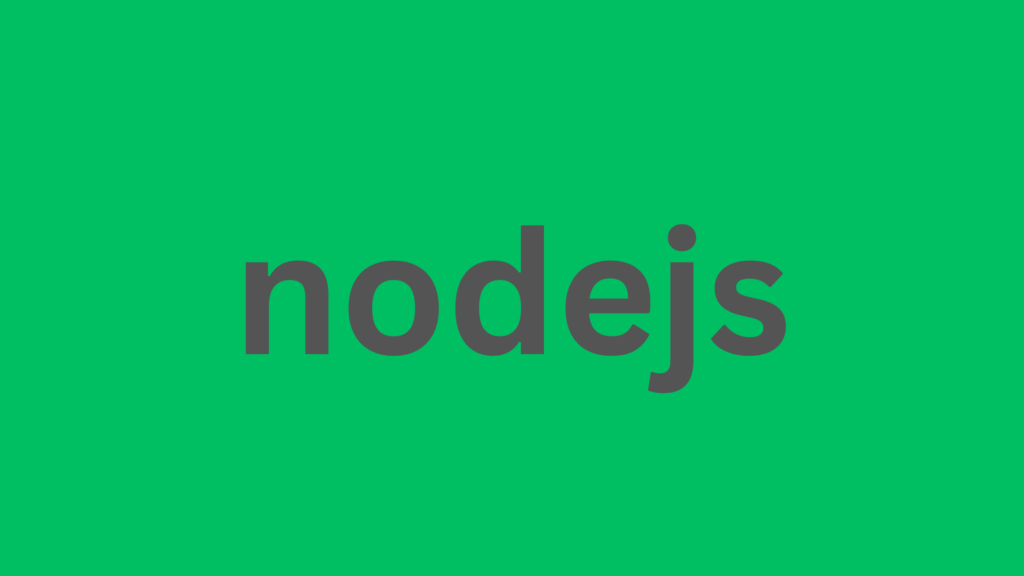
AI Meets Node.js: A Step-by-Step Guide to Boosting Productivity in VSCode
Visual Studio Code (VSCode) has become a go-to code editor for developers, and for good reason—it’s fast, extensible, and developer-friendly. Now, with the rise of AI-powered coding tools, you can supercharge your Node.js development workflow. These AI assistants, like GitHub Copilot, CodeGPT, and Tabnine, help you write cleaner code, suggest improvements, and speed up debugging. In this article, we’ll explore how to enable AI coding assistance in VSCode specifically tailored for Node.js projects.
Why Use AI Coding Assistance?
AI coding assistants bring several benefits:
- Improved Productivity: AI tools can auto-generate code snippets, saving you time.
- Error Reduction: AI can suggest fixes for bugs or issues in real-time.
- Code Suggestions: Get recommendations for best practices in Node.js.
- Learning Tool: For beginners, it’s a fantastic way to learn more about coding patterns.
Whether you’re a beginner or a seasoned Node.js developer, enabling AI assistance can significantly improve your efficiency.
Prerequisites
Before you enable AI assistance in VSCode, ensure you have the following:
- VSCode Installed: Download and install Visual Studio Code if you haven’t already.
- Node.js Installed: Install Node.js from nodejs.org.
- Extensions Marketplace Access: Make sure your VSCode installation can access the Extensions Marketplace.
- GitHub Account (for Copilot): Some AI tools, like GitHub Copilot, require a GitHub account.
Step 1: Choose the Right AI Extension
There are several AI-powered extensions available for VSCode. Here are some popular options:
- GitHub Copilot
- Developed by GitHub and OpenAI, GitHub Copilot is one of the most powerful tools for AI-assisted coding.
- It generates code snippets, suggests entire functions, and understands natural language prompts.
- Tabnine
- Tabnine focuses on contextual code completions and supports multiple programming languages, including JavaScript.
- It works offline, making it a privacy-conscious choice.
- CodeGPT
- CodeGPT integrates OpenAI’s GPT models directly into VSCode.
- Great for natural language queries and explanations.
- Kite (Optional)
- Kite offers AI-powered completions and documentation for JavaScript and Node.js.
Step 2: Install the AI Extension
Let’s walk through installing GitHub Copilot as an example:
- Open Extensions Marketplace:
- Launch VSCode and press
Ctrl+Shift+X
(orCmd+Shift+X
on macOS).
- Launch VSCode and press
- Search for GitHub Copilot:
- In the Extensions panel, type “GitHub Copilot” in the search bar.
- Install the Extension:
- Click the “Install” button for GitHub Copilot.
- Sign In with GitHub:
- After installation, sign in with your GitHub account to activate the extension.
- Enable Copilot:
- Follow the on-screen instructions to grant permissions and activate Copilot in your projects.
Step 3: Configure the Extension for Node.js
Once the AI extension is installed, configure it for optimal performance in Node.js projects.
- Create or Open a Node.js Project:
- Open your existing Node.js project or create a new one by running:bashCopyEdit
mkdir my-node-project cd my-node-project npm init -y
- Open your existing Node.js project or create a new one by running:bashCopyEdit
- Set Language Mode:
- Ensure that the file you are editing is in JavaScript or TypeScript mode.
- VSCode automatically detects the language based on the file extension (.js, .ts).
- Adjust Settings:
- Go to
File > Preferences > Settings
(orCode > Preferences > Settings
on macOS). - Search for the installed extension (e.g., “Copilot”) and tweak settings like:
- Auto-completion behavior
- Suggestions frequency
- Go to
Step 4: Test the AI Assistant
Now it’s time to test your AI-powered coding assistant:
- Start Coding:
- Open a
.js
file and start writing code. For example:javascriptCopyEditconst http = require('http');
- Watch as the AI suggests the next lines of code.
- Open a
- Use Natural Language Prompts:
- With GitHub Copilot or CodeGPT, you can type comments as prompts. For instance:javascriptCopyEdit
// Create an HTTP server that listens on port 3000
- The AI will generate relevant code based on your comment.
- With GitHub Copilot or CodeGPT, you can type comments as prompts. For instance:javascriptCopyEdit
- Review and Edit:
- While AI suggestions are helpful, always review the generated code for accuracy and security.
Troubleshooting Tips
- Extension Not Working? Ensure you’ve granted the necessary permissions and are signed in.
- Suggestions Not Relevant? Check if the language mode is set correctly in VSCode.
- Performance Issues? Disable unused extensions or increase memory allocation for VSCode.
Additional Tips for Node.js Developers
- Use AI tools to refactor existing code.
- Generate tests with AI assistance for better coverage.
- Experiment with different AI extensions to find the one that suits your style.
Conclusion
AI coding assistance in VSCode is a game-changer for Node.js developers. Whether you’re building a new app or maintaining an existing one, these tools can save time, improve code quality, and make coding more enjoyable. By following the steps outlined above, you can quickly enable and configure AI tools in your development environment.
So, why wait? Empower your Node.js projects with the magic of AI today!