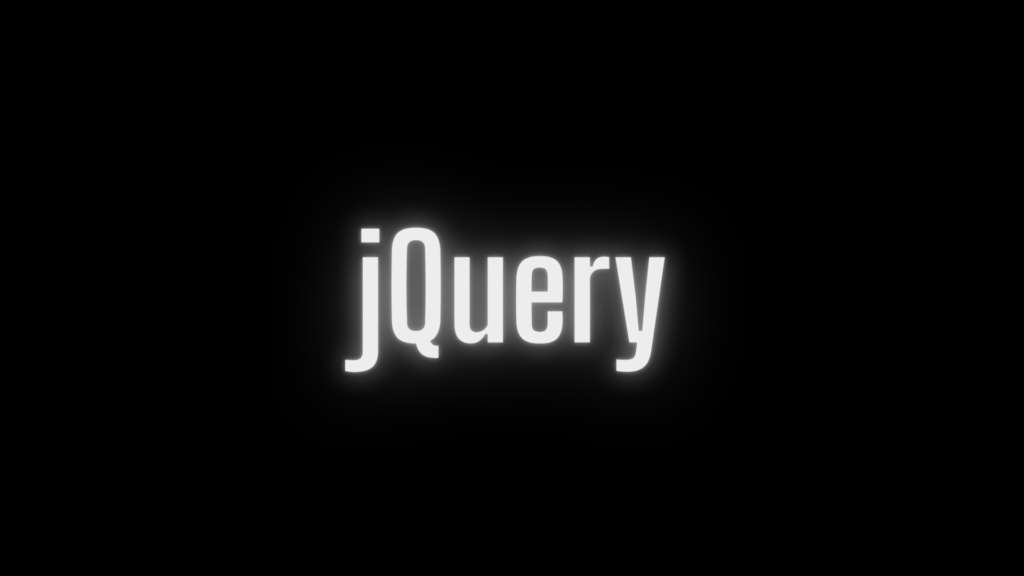
In web development, it’s common to want to check if an element is hidden or visible on a webpage. Whether you want to perform a certain action only if the element is visible or you need to determine whether the element’s styling is set to hide, jQuery provides an easy way to check the visibility of an element. In this article, we’ll explore how to check if an element is hidden in jQuery.
In jQuery, you can check if an element is hidden using the is()
method and the :hidden
selector.
Here’s an example:
if ($('#myElement').is(':hidden')) {
// The element is hidden
} else {
// The element is visible
}
In this example, #myElement
is the ID of the element you want to check. The is()
method checks if the element matches the selector :hidden
, which matches all elements that are currently hidden (with display: none
, visibility: hidden
, or an equivalent method).
If the element matches the :hidden
selector, the code inside the if
statement will run, indicating that the element is hidden. If the element is not hidden, the code inside the else
statement will run, indicating that the element is visible.
The :hidden
selector has been available in jQuery since version 1.0. The is()
method has also been available since version 1.0. Therefore, the syntax used in the example I provided should be available in any version of jQuery from 1.0 onwards.
How to Check if an Element is Hidden in Pure JavaScript?
In pure JavaScript, you can check if an element is hidden by using the getComputedStyle()
method to get the computed style of the element and then checking if the display
or visibility
property is set to none
.
Here’s an example:
const element = document.getElementById('myElement');
const styles = window.getComputedStyle(element);
if (styles.display === 'none' || styles.visibility === 'hidden') {
// The element is hidden
} else {
// The element is visible
}
In this example, myElement
is the ID of the element you want to check. The getComputedStyle()
method returns an object that represents the computed style of the element. You can then check if the display
or visibility
property of the computed style object is set to none
or hidden
, respectively. If the element is hidden, the code inside the if
statement will run, indicating that the element is hidden. If the element is not hidden, the code inside the else
statement will run, indicating that the element is visible.
Which Approach is better? jQuery or Pure JS?
Whether to use pure JavaScript or jQuery to check if an element is hidden depends on the context and requirements of your project.
If you are already using jQuery in your project, it may be more convenient to use the jQuery way of checking if an element is hidden since it’s a built-in feature of the library and can be accomplished with a single line of code.
On the other hand, if you’re not using jQuery or you want to minimize your reliance on external libraries, using pure JavaScript may be a better option. The pure JavaScript approach uses only native browser features, making it more lightweight and potentially faster to execute.
Ultimately, both approaches will achieve the same result, so it’s up to you to choose the one that best fits your project’s needs and constraints.