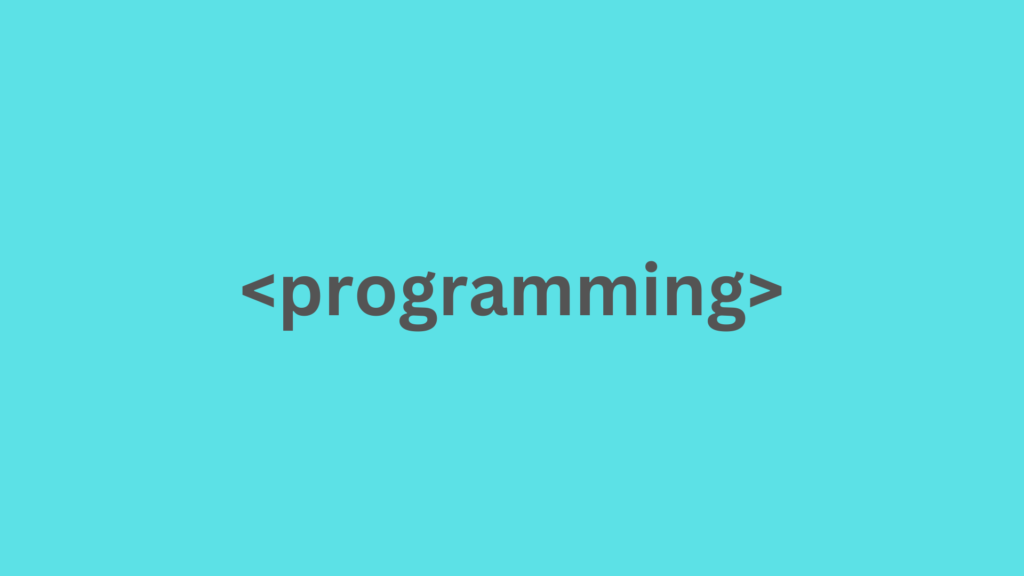
Python is a popular programming language that offers a wide range of functionalities and features to developers. One of the unique features of Python is the yield
keyword, which allows developers to create generators that can generate sequences of values on the fly, instead of computing them all at once and storing them in memory. Understanding the yield
keyword and how to use it can greatly enhance your Python programming skills and help you write more efficient and scalable code.
Understanding iterables and generators is important to understand the yield
keyword in Python because yield
is used to create generator functions, which are a type of iterable. Generator functions are functions that contain one or more yield
statements, which allow them to produce a sequence of values lazily, one at a time, as they are requested.
What are iterables in python?
In Python, an iterable is any object capable of returning its elements one at a time. Some examples of built-in Python iterables include lists, tuples, sets, and strings.
Iterables can be used in a for loop to iterate over each of their elements. For example, consider the following code:
my_list = [1, 2, 3, 4, 5] for element in my_list: print(element)
This code will iterate over each element in the my_list
list, printing each element to the console. This works because my_list
is an iterable object.
To be iterable, an object must implement the __iter__()
method, which returns an iterator object. An iterator is an object that produces the next value in the sequence when the __next__()
method is called on it. When there are no more values to produce, the iterator raises a StopIteration
exception.
Iterators can also be created using a function that contains one or more yield
statements. When the function is called, it returns a generator object, which is an iterator that produces values lazily.
In summary, an iterable in Python is any object capable of returning its elements one at a time, and it can be used in a for loop or other iterator-based constructs. Iterators are objects that produce the next value in the sequence when the __next__()
method is called, and they can be created using either the __iter__()
method or a function that contains one or more yield
statements.
What are generators in python ?
A generator is a type of iterable, like lists or tuples, but with a few key differences.
Generators are created using a function that contains one or more yield
statements. When the function is called, it returns a generator object, which can be iterated over using a for loop, or by calling the next() function on the generator object.
The primary difference between generators and other iterables is that generators produce a sequence of values lazily, i.e., they produce one value at a time, only when it is requested. This is in contrast to lists, which produce all their values at once and store them in memory.
This lazy evaluation makes generators highly memory-efficient, especially for large data sets or sequences of infinite length. Since generators only produce the values as they are needed, they can be more efficient than lists, which would have to store all the values in memory at once.
Another advantage of generators is that they can be infinite, meaning that they can produce a sequence of values without ever running out of values to produce. For example, a generator that produces an infinite sequence of even numbers would continue to produce values indefinitely.
In summary, generators in Python are a powerful tool for generating sequences of values, especially when working with large data sets or infinite sequences. They are created using functions that contain one or more yield
statements, and they produce values lazily, one at a time, as they are requested.
What does the “yield” keyword do in Python?
The yield
keyword is used in a function to create a generator. A generator is an iterable object that generates a sequence of values on the fly, instead of computing them all at once and storing them in memory.
When a function containing a yield
statement is called, it returns a generator object, which can be used to iterate over the sequence of values generated by the function. Each time the yield
statement is encountered, the function “pauses” and saves its state, so that it can be resumed later. When the generator’s __next__()
method is called, the function resumes execution from where it left off, continuing until the next yield
statement is encountered.
Here is an example of a generator function that generates a sequence of even numbers:
def generate_even_numbers(n): for i in range(n): if i % 2 == 0: yield i
When this function is called with an argument n
, it generates and returns a generator object. This generator can then be used to iterate over the sequence of even numbers generated by the function:
>>> gen = generate_even_numbers(10) >>> next(gen) 0 >>> next(gen) 2 >>> next(gen) 4 >>> list(gen) [6, 8]
Note that the yield
statement is used to generate the sequence of even numbers on the fly, and that the function’s state is saved between calls to next()
, allowing it to resume execution where it left off.
The yield
keyword in Python is a powerful tool that can greatly enhance your programming skills and help you write more efficient and scalable code. By allowing you to create generators that generate sequences of values on the fly, the yield
keyword can help you save memory and computational resources, making your code more efficient and performant. Understanding the yield
keyword and how to use it can be a valuable addition to your Python programming toolkit, and can help you tackle complex programming challenges with ease.