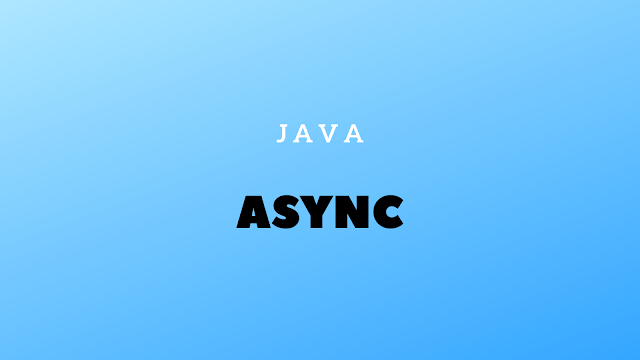
It is super easy to convert any remote call into an async call from Java 8 onwards. You can use a combination of java.util.concurrent.CompletableFuture and java.util.function.Supplier
This is a simple and non-invasive way to convert any existing code to async.
Java has predefined some of the functional interfaces that can simplify Lambda implementations for any application.
java.util.function.Supplier is a handy functional interface that can be implemented as a lambda for getting data from some remote source.
Below is a simple Java class that has a method that needs to be called Asynchrnously.
public class MyClass {
public Data getData() {
// some remote processing code
};
}
A simple blocking call would look like this
MyClass myobject = new MyClass();
Data dataObject = myobject.getData();
To invoke this method Asynchrnously you can use CompletableFuture.supplyAsync method as shown below code.
MyClass myobject = new MyClass();
CompletableFuture dataCompletableFuture = CompletableFuture.supplyAsync(() -> myobject.getData()); // this is async call that has a supplier Lambda
Data dataObject = dataCompletableFuture.get(); // this is a blocking call
Sequencing Multiple Calls Async Calls using CompletableFuture.thenCombine
Let us say we have 3 methods to call in our program. In a regular blocking call, it would look something like this.
MyClass myobject = new MyClass();
Data dataObject1 = myobject.getData1();
Data dataObject2 = myobject.getData2();
Data dataObject3 = myobject.getData3();
Aggregator aggregator = new Aggregator();
Data someDataCombined = aggregator.combine(dataObject1, dataObject2); // combines data of 2 objects
Data someDataCombined2 = aggregator.combine(someDataCombined, dataObject3); // combines data of 2 objects
public class Aggregator {
public combine(Data data1, Data data2) {
//some magic for aggregating data
}
}
To make the same three calls Asynchrnously you can use CompletableFuture.supplyAsync and CompletableFuture.thenCombine
MyClass myobject = new MyClass();
CompletableFuture dataCompletableFuture1 = CompletableFuture.supplyAsync(() -> myobject.getData1()); // first Async call
CompletableFuture dataCompletableFuture2 = CompletableFuture.supplyAsync(() -> myobject.getData2()); // second Async call
CompletableFuture dataCompletableFuture3 = CompletableFuture.supplyAsync(() -> myobject.getData3()); // third Async call
//Now the code to combine in sequence
dataCompletableFuture1
.thenCombine(dataCompletableFuture2, Aggregator::combine)
.thenCombine(dataCompletableFuture3, Aggregator::combine);