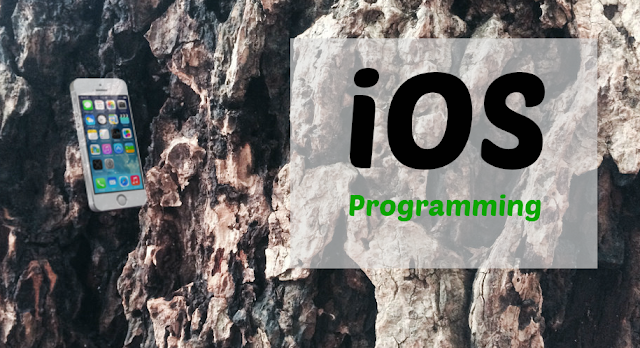
Creating a PDF file inside an iOS app may be easy however scaling the PDF file to right size may not be. An A4 sized PDF file is most common size and frequently used in printing documents.
The objective of this post is to explain how to create an A4-sized PDF file in iOS application from an HTML rendered UIWebView.
The basic class used in this task is explained below:
UIPrintPageRenderer
The object of this class is used to draw pages of content that are to be printed. This class typically overrides one or more draw methods from the below list:
- drawPageAtIndex:InRect:- It calls each of the other drawing methods in the order listed below.
- drawHeaderForPageAtIndex:InRect:- It is used to draw the content in the header.
- drawContentForPageAtIndex:InRect:- It is used to draw the content of the print job in the area between header and footer.
- drawFooterForPageAtIndex:InRect:- It is used to draw the content in the footer.
- drawPrintFormatter:ForPageAtIndex:- It is used to intermix the custom drawing with the drawing performed by the print formatter.
The below function is also used to carry out this task:
- UIGraphicsBeginPDFContextToData :- This function is used to create the PDF graphics context and associate with the destination for the PDF data.
- UIGraphicsGetPDFContextBounds :- It returns the current page bounds.
It’s enough with theory. Now lets take a look on the code to create PDF file fromUIWebView:
Step 1
First of all create an XCode project named CreatePDF and save it. This project will contain one ViewController. Take a UIWebView control on this ViewController and set its delegate to point to this ViewController. Also create UIWebView’s referencing outlet to interact with it.
Take a button on ViewController to create a PDF on its ‘Touch Up Inside’ event.
Step 2
Now load any URL in your UIWebView. For this you need to write the below code in ViewDidLoad:
[webView loadRequest:[NSURLRequest requestWithURL:[NSURL URLWithString:@"http://en.wikipedia.org/wiki/IOS "]]];
Here, ‘webView’ is the IBOUTLET of the UIWebView control. Now we have to wait till the page is fully loaded in the UIWebView. If we don’t wait till the page is loaded and try to create PDF file, then we will get an empty PDF file.
Step 3
After the page is fully loaded in UIWebView, call the below mentioned method from button’s touch up inside event:-(void)createPDFfromUIView:(NSString*)aFilename
{
int height, width, header, sidespace;
height = 1754;
width = 1240;
header = 15;
sidespace = 30;
// set header and footer spaces
UIEdgeInsets pageMargins = UIEdgeInsetsMake(header, sidespace, header, sidespace);
webView.viewPrintFormatter.contentInsets = pageMargins;
//Now create an object of UIPrintPageRenderer that is used to draw pages of content that are to be printed, with or without the assistance of print formatters.
UIPrintPageRenderer *renderer = [[UIPrintPageRenderer alloc] init];
[renderer addPrintFormatter:webView.viewPrintFormatter startingAtPageAtIndex:0];
CGSize pageSize = CGSizeMake(width, height);
CGRect printableRect = CGRectMake(pageMargins.left,
pageMargins.top,
pageSize.width - pageMargins.left - pageMargins.right,
pageSize.height - pageMargins.top - pageMargins.bottom);
CGRect paperRect = CGRectMake(0, 0, pageSize.width, pageSize.height);
[renderer setValue:[NSValue valueWithCGRect:paperRect] forKey:@"paperRect"];
[renderer setValue:[NSValue valueWithCGRect:printableRect] forKey:@"printableRect"];
//Now get an NSData object from UIPrintPageRenderer object with specified paper size.
NSData *pdfData = [self printToPDFWithRenderer: renderer paperRect:paperRect];
// save PDF file
NSString *saveFileName = [NSString stringWithFormat:@"%@%dx%d.pdf", aFilename, (int)pageSize.width, (int)pageSize.height];
NSArray* documentDirectories = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask,YES);
NSString* documentDirectory = [documentDirectories objectAtIndex:0];
NSString* savePath = [documentDirectory stringByAppendingPathComponent:saveFileName];
if([[NSFileManager defaultManager] fileExistsAtPath:savePath])
{
[[NSFileManager defaultManager] removeItemAtPath:savePath error:nil];
}
[pdfData writeToFile: savePath atomically: YES];
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil message:@"PDF File created and saved successfully." delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil, nil];
[alert show];
[activityView stopAnimating];
[activityView setHidden:YES];}
Step 4
In above method we have used a method named printToPDFWithRenderer to get the data from UIWebView. This method is described below:
- (NSData*) printToPDFWithRenderer:(UIPrintPageRenderer*)renderer paperRect:(CGRect)paperRect
{
NSMutableData *pdfData = [NSMutableData data];
UIGraphicsBeginPDFContextToData( pdfData, paperRect, nil );
[renderer prepareForDrawingPages: NSMakeRange(0, renderer.numberOfPages)];
CGRect bounds = UIGraphicsGetPDFContextBounds();
for ( int i = 0 ; i < renderer.numberOfPages ; i++ ) { UIGraphicsBeginPDFPage(); [renderer drawPageAtIndex:i inRect: bounds]; } UIGraphicsEndPDFContext(); return pdfData; }
Tejas Jasaniis a founder of Android Development Company named The APP Guruz, His major focus is on how to improve mobile users smart phone experience through development of mobile games and apps.